运用vue开发基础知识,实现添加学生5门科目的成绩,并计算出平均值,涉及到的姓名,班级用自己的信息,具体要求如下: 点击添加成绩,可从课程数据数组中,选择一门课添加,成绩随机生成一门成绩添加成功后,将本门课程从课程数组中删除,下次不可再选择!点击删除成绩,将本门课程重新添加至课程数字中,下次可添加该门课. 5072101 2 3 当课程数组中的课程全部添加完成后,提示,无课程添加: 5当页面中,没有成绩时,点击删除成绩提示,无成绩可删除
时间: 2023-07-31 14:09:45 浏览: 63
首先,在 Vue 中,我们需要定义一个数据对象,用来存储学生的信息和成绩。这个对象可以包括学生的姓名、班级、各门课程的成绩和平均分数。我们也需要定义一个课程数据数组,来存储可选择的课程。
```javascript
data() {
return {
student: {
name: '张三',
class: '一班',
courses: {
'语文': 0,
'数学': 0,
'英语': 0,
'物理': 0,
'化学': 0
},
average: 0
},
courseList: ['语文', '数学', '英语', '物理', '化学'],
selectedCourse: ''
}
}
```
接下来,我们需要实现添加成绩的功能。当用户点击“添加成绩”按钮时,我们需要从课程数据数组中随机选择一门课程,并生成一个随机成绩赋值给该门课程。然后,我们需要将该门课程从课程数据数组中删除,同时更新学生的成绩和平均分数。
```javascript
methods: {
addScore() {
if (this.courseList.length === 0) {
alert('无课程添加')
return
}
const randomIndex = Math.floor(Math.random() * this.courseList.length)
const selectedCourse = this.courseList[randomIndex]
const score = Math.floor(Math.random() * 101)
this.student.courses[selectedCourse] = score
this.student.average = this.calculateAverage()
this.courseList.splice(randomIndex, 1)
},
calculateAverage() {
const scores = Object.values(this.student.courses)
const sum = scores.reduce((a, b) => a + b, 0)
return parseFloat((sum / scores.length).toFixed(2))
}
}
```
最后,我们需要实现删除成绩的功能。当用户点击“删除成绩”按钮时,我们需要将该门课程重新添加到课程数据数组中,并清空该门课程的成绩。如果课程数据数组已经包含该门课程,则提示用户无法删除。
```javascript
deleteScore(course) {
if (this.courseList.includes(course)) {
alert('无成绩可删除')
return
}
this.courseList.push(course)
this.student.courses[course] = 0
this.student.average = this.calculateAverage()
}
```
在 HTML 中,我们可以使用 v-for 指令来循环渲染学生的成绩,并使用 v-on 指令来绑定事件处理函数。
```html
<table>
<thead>
<tr>
<th>姓名</th>
<th>班级</th>
<th v-for="course in Object.keys(student.courses)">{{ course }}</th>
<th>平均分</th>
</tr>
</thead>
<tbody>
<tr>
<td>{{ student.name }}</td>
<td>{{ student.class }}</td>
<td v-for="(score, course) in student.courses">{{ score }}</td>
<td>{{ student.average }}</td>
</tr>
</tbody>
</table>
<button @click="addScore">添加成绩</button>
<button v-for="course in courseList" :key="course" @click="selectedCourse = course">{{ course }}</button>
<button v-if="Object.values(student.courses).some(score => score > 0)" v-for="(score, course) in student.courses" :key="course" @click="deleteScore(course)">{{ course }} 删除成绩</button>
```
这样,我们就完成了添加学生成绩并计算平均值的功能。完整代码如下:
```javascript
<template>
<div>
<table>
<thead>
<tr>
<th>姓名</th>
<th>班级</th>
<th v-for="course in Object.keys(student.courses)">{{ course }}</th>
<th>平均分</th>
</tr>
</thead>
<tbody>
<tr>
<td>{{ student.name }}</td>
<td>{{ student.class }}</td>
<td v-for="(score, course) in student.courses">{{ score }}</td>
<td>{{ student.average }}</td>
</tr>
</tbody>
</table>
<button @click="addScore">添加成绩</button>
<button v-for="course in courseList" :key="course" @click="selectedCourse = course">{{ course }}</button>
<button v-if="Object.values(student.courses).some(score => score > 0)" v-for="(score, course) in student.courses" :key="course" @click="deleteScore(course)">{{ course }} 删除成绩</button>
</div>
</template>
<script>
export default {
data() {
return {
student: {
name: '张三',
class: '一班',
courses: {
'语文': 0,
'数学': 0,
'英语': 0,
'物理': 0,
'化学': 0
},
average: 0
},
courseList: ['语文', '数学', '英语', '物理', '化学'],
selectedCourse: ''
}
},
methods: {
addScore() {
if (this.courseList.length === 0) {
alert('无课程添加')
return
}
const randomIndex = Math.floor(Math.random() * this.courseList.length)
const selectedCourse = this.courseList[randomIndex]
const score = Math.floor(Math.random() * 101)
this.student.courses[selectedCourse] = score
this.student.average = this.calculateAverage()
this.courseList.splice(randomIndex, 1)
},
calculateAverage() {
const scores = Object.values(this.student.courses)
const sum = scores.reduce((a, b) => a + b, 0)
return parseFloat((sum / scores.length).toFixed(2))
},
deleteScore(course) {
if (this.courseList.includes(course)) {
alert('无成绩可删除')
return
}
this.courseList.push(course)
this.student.courses[course] = 0
this.student.average = this.calculateAverage()
}
}
}
</script>
```
阅读全文
相关推荐
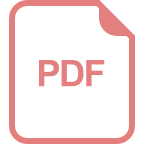
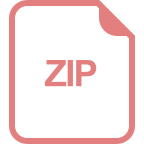
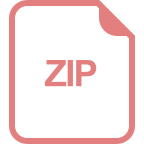
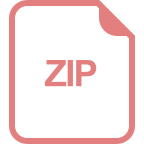
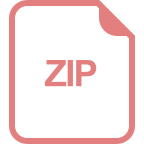
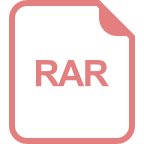
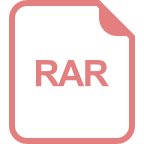
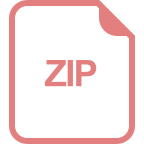
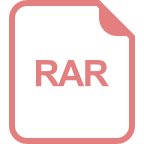
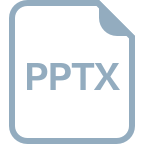
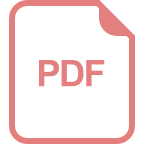
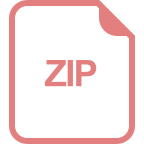
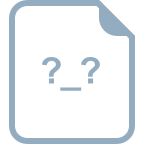
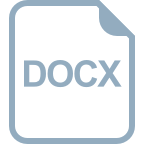
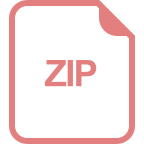
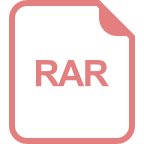
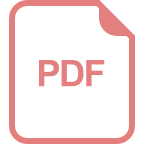