python将多个点坐标进行两两组合
时间: 2023-09-10 08:05:56 浏览: 242
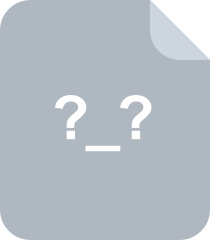
python将两个数组合并成一个

您可以使用`itertools`模块中的`combinations`函数来实现将多个点坐标进行两两组合的操作。具体代码如下:
```python
import itertools
points = [(1, 2), (3, 4), (5, 6), (7, 8)]
combinations = list(itertools.combinations(points, 2))
print(combinations)
```
输出结果为:
```
[((1, 2), (3, 4)), ((1, 2), (5, 6)), ((1, 2), (7, 8)), ((3, 4), (5, 6)), ((3, 4), (7, 8)), ((5, 6), (7, 8))]
```
其中,`combinations`变量存储了所有的两两组合结果。每个组合都是一个由两个点坐标组成的元组。
阅读全文
相关推荐
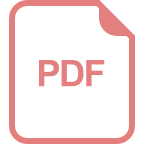
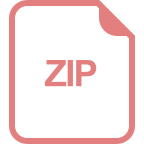


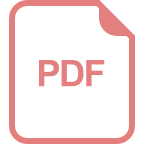
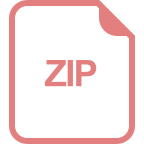
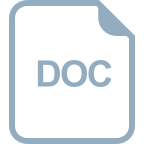
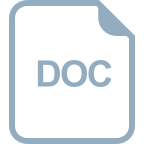
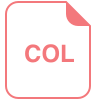
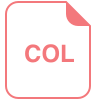
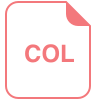
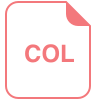
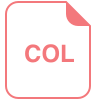
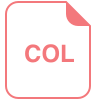
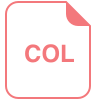


