spring整合mybatis纯注解
时间: 2023-04-14 13:02:55 浏览: 105
Spring整合MyBatis可以使用纯注解的方式,具体步骤如下:
1. 在Spring配置文件中配置数据源和事务管理器。
2. 在MyBatis的Mapper接口上使用@Mapper注解,表示这是一个Mapper接口。
3. 在Mapper接口的方法上使用@Select、@Insert、@Update、@Delete等注解,表示对应的SQL语句。
4. 在Spring配置文件中配置MapperScannerConfigurer,指定Mapper接口所在的包路径。
5. 在Service层中注入Mapper接口,即可使用Mapper接口中定义的方法进行数据库操作。
示例代码如下:
1. Spring配置文件中配置数据源和事务管理器
```xml
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/test"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</bean>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
```
2. 在Mapper接口上使用@Mapper注解
```java
@Mapper
public interface UserMapper {
@Select("select * from user where id=#{id}")
User getUserById(int id);
@Insert("insert into user(name, age) values(#{name}, #{age})")
int addUser(User user);
@Update("update user set name=#{name}, age=#{age} where id=#{id}")
int updateUser(User user);
@Delete("delete from user where id=#{id}")
int deleteUser(int id);
}
```
3. 在Spring配置文件中配置MapperScannerConfigurer
```xml
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.example.mapper"/>
</bean>
```
4. 在Service层中注入Mapper接口
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserById(int id) {
return userMapper.getUserById(id);
}
@Override
public int addUser(User user) {
return userMapper.addUser(user);
}
@Override
public int updateUser(User user) {
return userMapper.updateUser(user);
}
@Override
public int deleteUser(int id) {
return userMapper.deleteUser(id);
}
}
```
阅读全文
相关推荐
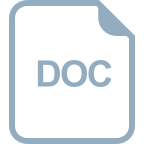
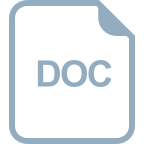
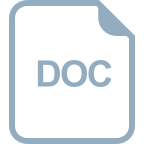
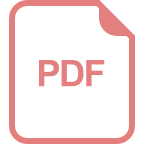
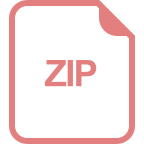


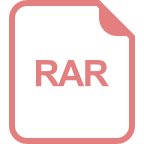
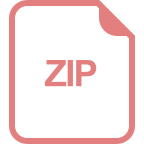
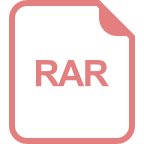
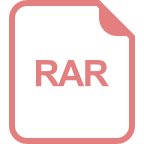
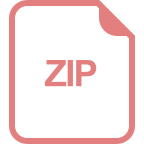
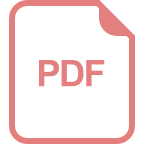
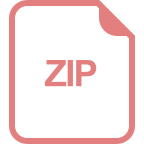
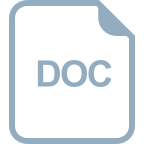
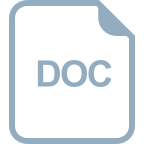
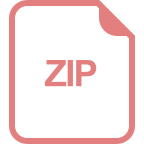