java编写一个生产者消费者队列
时间: 2024-05-14 12:12:50 浏览: 77
这里是一个简单的生产者消费者队列的Java实现:
```
import java.util.LinkedList;
import java.util.Queue;
public class ProducerConsumerQueue {
private final Queue<Integer> queue = new LinkedList<>();
private final int MAX_SIZE = 10;
public void produce() {
int i = 0;
while (i < 20) { // 生产20个物品
synchronized (queue) {
while (queue.size() == MAX_SIZE) { // 如果队列已满,等待消费者消费
try {
queue.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
queue.add(i);
System.out.println("Produced " + i);
i++;
queue.notifyAll(); // 唤醒所有等待的消费者
}
}
}
public void consume() {
int i = 0;
while (i < 20) { // 消费20个物品
synchronized (queue) {
while (queue.isEmpty()) { // 如果队列为空,等待生产者生产
try {
queue.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
int num = queue.remove();
System.out.println("Consumed " + num);
i++;
queue.notifyAll(); // 唤醒所有等待的生产者
}
}
}
public static void main(String[] args) {
ProducerConsumerQueue queue = new ProducerConsumerQueue();
Thread producerThread = new Thread(queue::produce);
Thread consumerThread = new Thread(queue::consume);
producerThread.start();
consumerThread.start();
}
}
```
在这个实现中,生产者负责生产物品并将其添加到队列中,消费者负责从队列中取出物品并消费。如果队列已满,生产者将等待消费者消费;如果队列为空,消费者将等待生产者生产。使用synchronized关键字和wait()、notifyAll()方法来实现同步和线程通信。
阅读全文
相关推荐
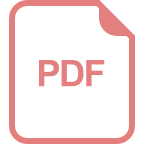
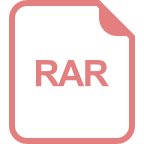
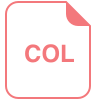

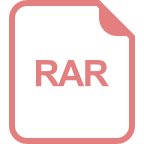
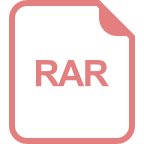
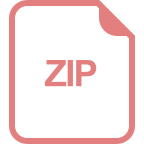
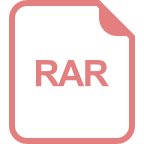
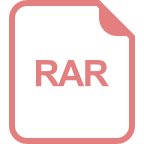
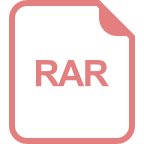
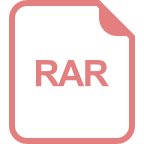
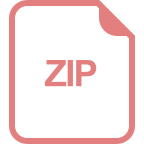
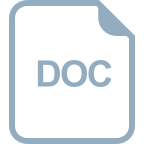
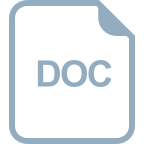
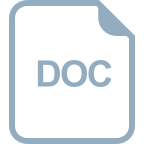
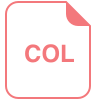
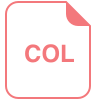