用c++写一个游乐园系统,游客需要注册,登录,充值后买票,票的种类为单人票,团购票和月票,买完票后提示顾客余额,并计算游乐场一天的营业额
时间: 2023-10-21 17:07:16 浏览: 61
好的,这是一个比较复杂的系统,需要细心编写,以下是一个简单的实现,可能还有些不完善的地方,仅供参考。
```
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class User {
public:
User(string name, string password) : name(name), password(password), balance(0) {}
string getName() { return name; }
void setBalance(double balance) { this->balance = balance; }
double getBalance() { return balance; }
bool checkPassword(string password) { return this->password == password; }
private:
string name;
string password;
double balance;
};
class Ticket {
public:
Ticket(string type, double price) : type(type), price(price), count(0) {}
string getType() { return type; }
double getPrice() { return price; }
int getCount() { return count; }
void addCount(int count) { this->count += count; }
private:
string type;
double price;
int count;
};
class PlayGround {
public:
PlayGround() {
users.push_back(User("admin", "admin"));
tickets.push_back(Ticket("single", 50));
tickets.push_back(Ticket("group", 180));
tickets.push_back(Ticket("monthly", 350));
}
bool addUser(string name, string password) {
for (auto user : users) {
if (user.getName() == name) {
return false;
}
}
users.push_back(User(name, password));
return true;
}
bool login(string name, string password) {
for (auto user : users) {
if (user.getName() == name && user.checkPassword(password)) {
currentUser = user;
return true;
}
}
return false;
}
bool recharge(double amount) {
if (currentUser.getBalance() + amount < 0) {
return false;
}
currentUser.setBalance(currentUser.getBalance() + amount);
return true;
}
bool buyTicket(string type, int count) {
for (auto ticket : tickets) {
if (ticket.getType() == type) {
double totalPrice = ticket.getPrice() * count;
if (currentUser.getBalance() < totalPrice) {
return false;
}
ticket.addCount(count);
currentUser.setBalance(currentUser.getBalance() - totalPrice);
return true;
}
}
return false;
}
void displayBalance() {
cout << "Your balance: " << currentUser.getBalance() << endl;
}
void displayDailyIncome() {
double totalIncome = 0;
for (auto ticket : tickets) {
totalIncome += ticket.getPrice() * ticket.getCount();
}
cout << "Daily income: " << totalIncome << endl;
}
private:
vector<User> users;
vector<Ticket> tickets;
User currentUser;
};
int main() {
PlayGround playGround;
string name, password;
cout << "Please register:" << endl;
cout << "Name: ";
cin >> name;
cout << "Password: ";
cin >> password;
if (!playGround.addUser(name, password)) {
cout << "Register failed, the name has been used." << endl;
return 0;
}
cout << "Register succeeded." << endl;
cout << "Please login:" << endl;
while (true) {
cout << "Name: ";
cin >> name;
cout << "Password: ";
cin >> password;
if (playGround.login(name, password)) {
cout << "Login succeeded." << endl;
break;
}
cout << "Login failed, please try again." << endl;
}
while (true) {
int choice;
cout << "1. Recharge" << endl;
cout << "2. Buy ticket" << endl;
cout << "3. Display balance" << endl;
cout << "4. Display daily income" << endl;
cout << "5. Exit" << endl;
cout << "Please choose: ";
cin >> choice;
switch (choice) {
case 1:
double amount;
cout << "Please input the amount: ";
cin >> amount;
if (playGround.recharge(amount)) {
cout << "Recharge succeeded." << endl;
}
else {
cout << "Recharge failed, please try again." << endl;
}
break;
case 2:
string type;
int count;
cout << "Please choose ticket type (single/group/monthly): ";
cin >> type;
cout << "Please input count: ";
cin >> count;
if (playGround.buyTicket(type, count)) {
cout << "Buy ticket succeeded." << endl;
}
else {
cout << "Buy ticket failed, please try again." << endl;
}
break;
case 3:
playGround.displayBalance();
break;
case 4:
playGround.displayDailyIncome();
break;
case 5:
return 0;
default:
cout << "Invalid choice, please try again." << endl;
}
}
}
```
注意:以上代码只是一个简单的实现,可能还有一些不完善的地方,需要根据实际情况进行修改和完善。
阅读全文
相关推荐
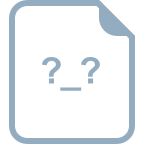
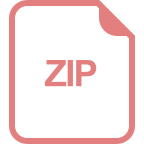
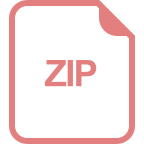
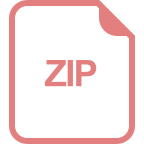
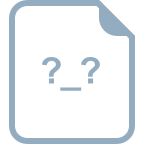
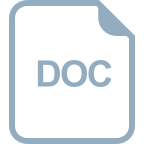
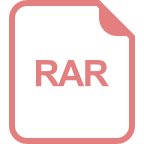
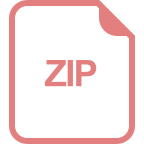
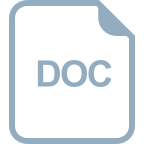