php调用windows的cmd命令
时间: 2023-08-10 17:57:32 浏览: 62
你可以使用PHP的`exec()`函数来调用Windows的cmd命令。`exec()`函数允许你在PHP脚本中执行外部命令,并返回命令的输出。
下面是一个简单的示例,演示如何使用`exec()`函数调用Windows的cmd命令:
```php
$command = 'dir'; // 要执行的命令
$output = array(); // 存储命令输出的数组
// 执行命令
exec($command, $output);
// 打印输出结果
foreach ($output as $line) {
echo $line . "<br>";
}
```
在上面的示例中,我们使用`dir`命令列出当前目录的文件和文件夹。你可以根据自己的需要替换`$command`变量为你想要执行的任何Windows cmd命令。
请注意,使用`exec()`函数调用外部命令可能存在安全风险,请确保你只执行可信任的命令,并对用户输入进行适当的验证和过滤。
相关问题
unity调用cmd命令
在Unity中,可以使用System.Diagnostics.Process类来调用cmd命令。以下是一个示例代码,该代码将在Windows系统中打开命令提示符并运行dir命令:
```
using System.Diagnostics;
public class CmdTest : MonoBehaviour
{
void Start()
{
Process process = new Process();
process.StartInfo.FileName = "cmd.exe";
process.StartInfo.Arguments = "/c dir";
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.Start();
string output = process.StandardOutput.ReadToEnd();
Debug.Log(output);
process.WaitForExit();
}
}
```
该代码中,首先创建一个Process对象并指定要运行的命令提示符程序(cmd.exe),然后设置命令行参数(/c dir)。启用RedirectStandardOutput属性后,可以读取命令提示符的输出。最后,等待命令提示符进程退出。
java调用cmd命令
Java可以通过`Runtime.getRuntime().exec()`方法来执行命令行命令。例如,要在Java中运行"dir"命令来列出当前目录下的文件,可以这样做:
```java
try {
Process p = Runtime.getRuntime().exec("cmd /c dir");
BufferedReader reader = new BufferedReader(new InputStreamReader(p.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
```
在这个例子中,我们使用`Runtime.getRuntime().exec()`方法来运行"cmd /c dir"命令,并使用`BufferedReader`从命令的输出中读取数据。最后,我们将输出打印到控制台上。
需要注意的是,在执行命令时,需要指定`cmd /c`前缀,它告诉系统要使用命令行解释器来执行命令。
相关推荐
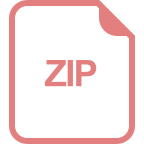











