java oss文件下载进度条
时间: 2023-10-14 22:27:04 浏览: 183
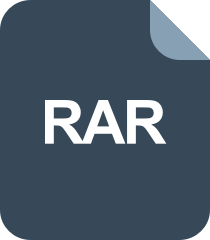
带进度条的文件下载

你可以使用阿里云的Java SDK来实现OSS文件下载,并且通过多线程和进度条来显示下载进度。以下是一个示例代码:
```
import com.aliyun.oss.OSS;
import com.aliyun.oss.event.ProgressEvent;
import com.aliyun.oss.event.ProgressEventType;
import com.aliyun.oss.event.ProgressListener;
import com.aliyun.oss.model.GetObjectRequest;
import com.aliyun.oss.model.OSSObject;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class OSSDownloadWithProgress {
private static final String ACCESS_KEY_ID = "<yourAccessKeyId>";
private static final String ACCESS_KEY_SECRET = "<yourAccessKeySecret>";
private static final String ENDPOINT = "<yourEndpoint>";
private static final String BUCKET_NAME = "<yourBucketName>";
private static final String OBJECT_KEY = "<yourObjectKey>";
private static final String DOWNLOAD_FILE_NAME = "<yourDownloadFileName>";
private static final int THREAD_POOL_SIZE = 5;
public static void main(String[] args) {
// 创建OSSClient实例
OSS ossClient = new OSSClientBuilder().build(ENDPOINT, ACCESS_KEY_ID, ACCESS_KEY_SECRET);
// 创建线程池
ExecutorService executorService = Executors.newFixedThreadPool(THREAD_POOL_SIZE);
// 创建GetObjectRequest对象并设置进度监听器
GetObjectRequest getObjectRequest = new GetObjectRequest(BUCKET_NAME, OBJECT_KEY);
getObjectRequest.setProgressListener(new ProgressListener() {
private long bytesWritten = 0;
private long totalBytes = -1;
private boolean succeed = false;
@Override
public void progressChanged(ProgressEvent progressEvent) {
long bytes = progressEvent.getBytes();
ProgressEventType eventType = progressEvent.getEventType();
switch (eventType) {
case TRANSFER_STARTED_EVENT:
System.out.println("Start to download...");
break;
case RESPONSE_CONTENT_LENGTH_EVENT:
this.totalBytes = bytes;
System.out.println(this.totalBytes + " bytes in total will be downloaded to a local file");
break;
case RESPONSE_BYTE_TRANSFER_EVENT:
this.bytesWritten += bytes;
if (this.totalBytes != -1) {
int percent = (int) (this.bytesWritten * 100.0 / this.totalBytes);
System.out.println(bytes + " bytes have been written at this time, download progress: " + percent + "% (" + this.bytesWritten + "/" + this.totalBytes + ")");
} else {
System.out.println(bytes + " bytes have been written at this time, download ratio: unknown" + "(" + this.bytesWritten + "/...)");
}
break;
case TRANSFER_COMPLETED_EVENT:
this.succeed = true;
System.out.println("Succeed to download, " + this.bytesWritten + " bytes have been transferred in total");
break;
case TRANSFER_FAILED_EVENT:
System.out.println("Failed to download, " + this.bytesWritten + " bytes have been transferred");
break;
default:
break;
}
}
});
// 提交下载任务到线程池
executorService.submit(() -> {
try {
// 下载OSS文件到本地
OSSObject ossObject = ossClient.getObject(getObjectRequest);
BufferedInputStream in = new BufferedInputStream(ossObject.getObjectContent());
File file = new File(DOWNLOAD_FILE_NAME);
FileOutputStream out = new FileOutputStream(file);
byte[] buffer = new byte[8192];
int len;
while ((len = in.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
out.close();
in.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
// 关闭OSSClient和线程池
ossClient.shutdown();
executorService.shutdown();
}
});
}
}
```
在上面的代码中,我们通过创建GetObjectRequest对象并设置进度监听器来实现OSS文件的下载进度显示。然后,我们将下载任务提交到线程池中,并在下载完成后关闭OSSClient和线程池。
阅读全文
相关推荐




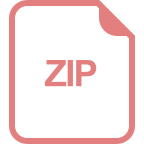
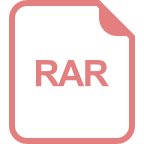
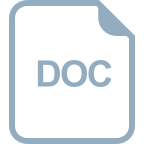
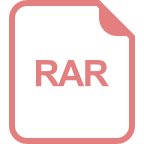
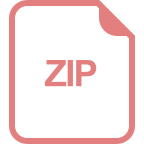
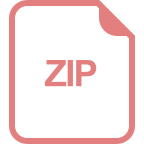
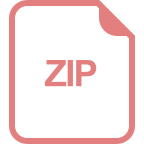
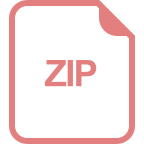
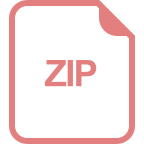
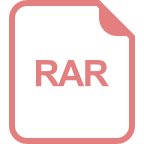
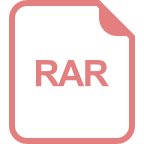