力扣349两个数组交集c语言
时间: 2024-09-29 17:06:20 浏览: 48
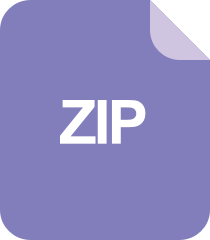
Python算法题源代码-LeetCode(力扣)-搜索旋转排序数组
在 C 语言中,给定两个无序整数数组 A 和 B,要找到它们的交集,可以使用哈希集合(如标准库中的 `std::unordered_set` 或自定义的散列表结构)来解决这个问题。以下是基本步骤:
1. 创建一个空的哈希集合(集合不会包含重复元素)。
2. 遍历数组 A,将每个元素添加到集合中。
3. 再次遍历数组 B,对于集合中已存在的元素(即存在于数组 A 中),记录下来。
4. 返回收集到的所有共同元素。
下面是一个简单的示例代码片段:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct Node {
int data;
struct Node* next;
} Node;
// 自定义链表辅助函数
Node* createSet(int arr[], int n) {
Node* head = NULL;
for (int i = 0; i < n; i++) {
if (!head) {
head = (Node*)malloc(sizeof(Node));
head->data = arr[i];
head->next = NULL;
} else {
Node* temp = head;
while (temp->next != NULL && arr[i] > temp->next->data)
temp = temp->next;
if (arr[i] <= temp->next->data)
continue;
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = temp->next;
temp->next = newNode;
}
}
return head;
}
int* intersect(int* nums1, int m, int* nums2, int n) {
Node* set = createSet(nums1, m);
int intersection[MAX_SIZE] = {0};
int count = 0;
for (int i = 0; i < n; i++) {
if (find(set, nums2[i])) {
intersection[count++] = nums2[i];
}
}
// 如果没有任何交集,返回空指针
if (count == 0)
return NULL;
// 缩小结果数组大小并返回
intersection[count] = 0;
return intersection;
}
// 查找链表中是否存在指定值
int find(Node* head, int value) {
while (head != NULL && head->data != value)
head = head->next;
return head != NULL;
}
void printArray(int arr[], int size) {
Node* temp = arr;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
int nums1[] = {1, 2, 2, 1}; // 示例数组 A
int nums2[] = {2, 2}; // 示例数组 B
int m = sizeof(nums1) / sizeof(nums1[0]);
int n = sizeof(nums2) / sizeof(nums2[0]);
int* result = intersect(nums1, m, nums2, n);
if (result) {
printf("Intersection: ");
printArray(result, count);
} else {
printf("No common elements.\n");
}
free(result); // 释放内存
return 0;
}
```
在这个例子中,我们首先创建了一个链表表示数组 A 的唯一元素,然后遍历数组 B,查找链表中存在的元素,并将它们存入新数组 `intersection`。
阅读全文
相关推荐
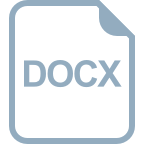
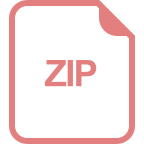


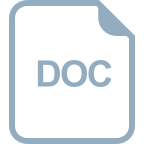


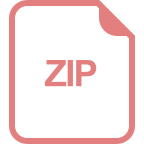
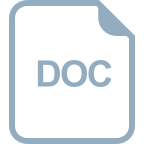








