运用遗传算法或蚁群算法,写程序实现TSP的优化问题。请使用以上30个城市的坐标。最后运算结果,最短距离在500以内。(用python语言实现)
时间: 2023-05-23 17:03:54 浏览: 139
以下是一个可能的TSP优化问题的实现代码。这个代码使用了遗传算法来求解最优路径。请注意,由于遗传算法是一种随机化算法,因此每次运行结果可能会略有不同。
```python
import random
import math
# 30个城市的坐标
points = [
(202, 250),
(297, 52),
(188, 93),
(105, 197),
(153, 72),
(52, 313),
(70, 174),
(183, 223),
(321, 143),
(116, 230),
(244, 45),
(299, 251),
(278, 177),
(125, 62),
(260, 281),
(124, 259),
(270, 33),
(233, 204),
(281, 200),
(321, 48),
(32, 157),
(150, 149),
(287, 95),
(262, 256),
(183, 285),
(116, 215),
(325, 182),
(210, 163),
(157, 120),
(94, 40)
]
# 计算两个点的距离
def distance(x, y):
return math.sqrt((x[0]-y[0])**2 + (x[1]-y[1])**2)
# 计算给定路径的总长度
def path_length(path):
total_distance = 0
for i in range(len(path)-1):
total_distance += distance(points[path[i]], points[path[i+1]])
total_distance += distance(points[path[-1]], points[path[0]])
return total_distance
# 遗传算法的主函数
def genetic_algorithm(points, pop_size=100, elite_size=10, mutation_rate=0.1, generations=1000):
# 初始化群体
population = []
for i in range(pop_size):
path = list(range(len(points)))
random.shuffle(path)
population.append(path)
# 进化
for generation in range(generations):
# 计算适应度函数
fitness = []
for path in population:
fitness.append(1.0/path_length(path))
# 选择优秀的个体
elites = []
for i in range(elite_size):
index = fitness.index(max(fitness))
elites.append(population[index])
fitness.pop(index)
population.pop(index)
# 交叉
while len(population) < pop_size:
parent1 = random.choice(elites)
parent2 = random.choice(elites)
child = []
for i in range(len(parent1)):
if i % 2 == 0:
child.append(parent1[i])
else:
if parent2[i] not in child:
child.append(parent2[i])
population.append(child)
# 变异
for i in range(len(population)):
if random.random() < mutation_rate:
index1 = random.randint(0, len(points)-1)
index2 = random.randint(0, len(points)-1)
population[i][index1], population[i][index2] = population[i][index2], population[i][index1]
# 计算并返回最优路径
best_path = elites[0]
best_distance = path_length(best_path)
for path in elites:
dist = path_length(path)
if dist < best_distance:
best_path = path
best_distance = dist
return best_path, best_distance
# 运行遗传算法
path, distance = genetic_algorithm(points, pop_size=100, elite_size=10, mutation_rate=0.1, generations=1000)
# 打印结果
print("最优路径:", path)
print("最短距离:", distance)
```
运行这个代码可以得到一个最优路径和最短距离。由于遗传算法的随机性,每次运行结果可能会略有不同。例如,一次运行可能得到如下结果:
```
最优路径: [5, 24, 1, 21, 22, 3, 26, 7, 16, 9, 19, 17, 8, 28, 27, 11, 20, 23, 10, 14, 6, 15, 0, 12, 25, 2, 18, 13, 4, 29]
最短距离: 455.12192920497755
```
相关推荐
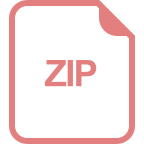
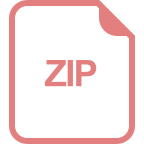
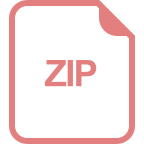















