用python设计一个带有纹理贴图的六面体,在空中一边自传一边沿椐圆轨道绕地球运行的过程
时间: 2024-03-14 16:47:50 浏览: 56
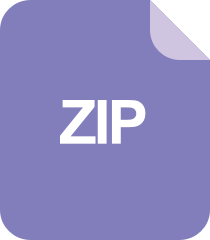
OpenGL绘制旋转六面体并纹理贴图.zip

好的,我可以为您提供一些思路和代码示例。
首先,我们需要安装一些必要的库,如pygame、numpy和pyopengl。可以通过以下命令来安装:
```python
pip install pygame numpy pyopengl
```
接下来,我们可以开始编写代码。以下是实现您所需功能的基本框架:
```python
import pygame
import numpy as np
from OpenGL.GL import *
from OpenGL.GLU import *
# 窗口大小
WIDTH = 800
HEIGHT = 600
# 地球半径
EARTH_RADIUS = 6.371 * 10 ** 6 # 单位:米
# 六面体边长
CUBE_SIDE = 1
# 六面体顶点坐标
vertices = np.array([...])
# 六面体面的顶点索引
faces = np.array([...])
# 纹理坐标
tex_coords = np.array([...])
# 纹理图片路径
texture_path = "texture.jpg"
# 圆轨道参数
orbit_radius = 10 * EARTH_RADIUS # 轨道半径
orbit_speed = 0.01 # 自传速度和公转速度
self_rotation_speed = 0.01
def load_texture(texture_path):
"""加载纹理图片并返回纹理ID"""
texture_surface = pygame.image.load(texture_path)
texture_data = pygame.image.tostring(texture_surface, "RGB", 1)
width = texture_surface.get_width()
height = texture_surface.get_height()
texture_id = glGenTextures(1)
glBindTexture(GL_TEXTURE_2D, texture_id)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, width, height, 0, GL_RGB, GL_UNSIGNED_BYTE, texture_data)
return texture_id
def draw_cube(texture_id):
"""绘制带纹理的六面体"""
glEnable(GL_TEXTURE_2D)
glBindTexture(GL_TEXTURE_2D, texture_id)
glBegin(GL_TRIANGLES)
for face in faces:
for vertex_index in face:
glTexCoord2fv(tex_coords[vertex_index])
glVertex3fv(vertices[vertex_index])
glEnd()
glDisable(GL_TEXTURE_2D)
def draw_orbit():
"""绘制轨道"""
glPushMatrix()
glColor3f(1.0, 1.0, 1.0)
glBegin(GL_LINE_LOOP)
for i in range(100):
angle = 2 * np.pi * i / 100
x = orbit_radius * np.cos(angle)
y = orbit_radius * np.sin(angle)
glVertex3f(x, y, 0)
glEnd()
glPopMatrix()
def main():
pygame.init()
pygame.display.set_mode((WIDTH, HEIGHT), pygame.OPENGL | pygame.DOUBLEBUF)
glEnable(GL_DEPTH_TEST)
glEnable(GL_LIGHTING)
glEnable(GL_LIGHT0)
glLightfv(GL_LIGHT0, GL_POSITION, [0, 0, 1, 0])
glMatrixMode(GL_PROJECTION)
gluPerspective(45.0, WIDTH / HEIGHT, 0.1, 100.0)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
gluLookAt(0, -20, 0, 0, 0, 0, 0, 0, 1)
texture_id = load_texture(texture_path)
angle = 0
orbit_angle = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glPushMatrix()
glRotatef(angle, 1, 0, 0)
glRotatef(orbit_angle, 0, 0, 1)
draw_cube(texture_id)
glPopMatrix()
draw_orbit()
angle += self_rotation_speed
orbit_angle += orbit_speed
pygame.display.flip()
if __name__ == "__main__":
main()
```
上面的代码中,`vertices`、`faces`和`tex_coords`分别表示六面体的顶点坐标、面的顶点索引和纹理坐标。您需要根据您所需的六面体的形状自行定义这些参数。`load_texture`函数用于加载纹理图片并返回纹理ID。`draw_cube`函数用于绘制带纹理的六面体。`draw_orbit`函数用于绘制轨道。`main`函数是主循环函数。
在主循环中,我们使用`glRotatef`函数来实现自传和公转。`angle`表示当前六面体自传的角度,`orbit_angle`表示当前六面体沿轨道公转的角度。`self_rotation_speed`和`orbit_speed`分别表示自传速度和公转速度。
最后,您需要将代码中的`vertices`、`faces`、`tex_coords`和`texture_path`替换为您自己的参数,并根据需要调整其他参数。
阅读全文
相关推荐
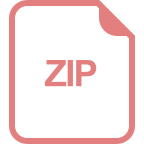
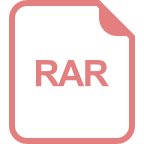




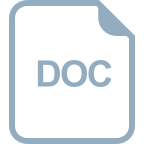
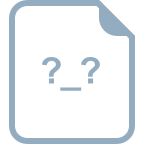
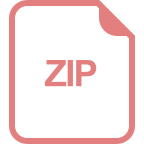
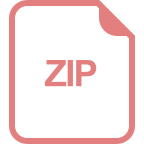
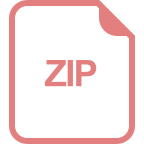