es6工厂模式,使用es6语法写出一个demo
时间: 2023-11-19 13:07:10 浏览: 143
当我们使用 ES6 语法时,通常会使用 Class 关键字来声明一个类,然后使用 constructor 方法定义类的构造函数,接着在类中定义方法。ES6 的类不仅可以用于创建对象,还可以用于创建工厂。下面就是一个使用 ES6 语法实现的工厂模式的示例代码:
```javascript
class Animal {
constructor(name, age, species) {
this.name = name;
this.age = age;
this.species = species;
}
makeSound() {
console.log(`The ${this.species} ${this.name} is making a sound.`);
}
}
class AnimalFactory {
createAnimal(name, age, species) {
return new Animal(name, age, species);
}
}
const factory = new AnimalFactory();
const animal1 = factory.createAnimal('Lion', 2, 'Mammal');
const animal2 = factory.createAnimal('Eagle', 3, 'Bird');
animal1.makeSound(); // The Mammal Lion is making a sound.
animal2.makeSound(); // The Bird Eagle is making a sound.
```
在上面的代码中,我们首先定义了一个 Animal 类,它有三个属性:name、age 和 species。然后在类中定义了一个 makeSound 方法,用于输出动物正在发出声音的信息。
接着我们定义了一个 AnimalFactory 类,它有一个 createAnimal 方法,用于创建 Animal 对象。最后我们通过实例化 AnimalFactory 类来创建两个 Animal 对象,并调用它们的 makeSound 方法来输出信息。
这就是一个使用 ES6 语法实现的工厂模式的示例代码。
阅读全文
相关推荐
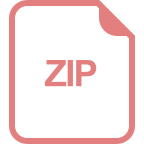
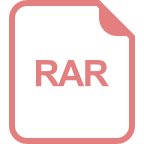
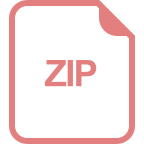
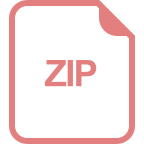
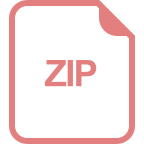
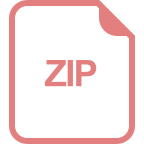
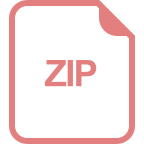
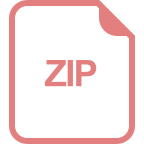
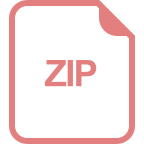
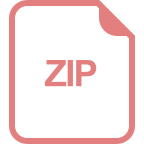
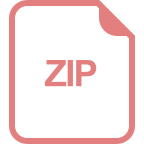
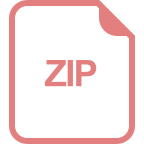
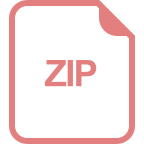
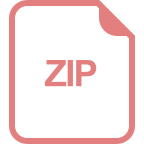
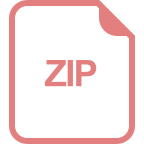
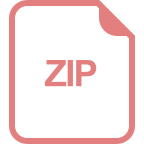
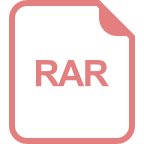