写一段android 使用fragmeng切换界面的代码
时间: 2024-05-02 15:17:59 浏览: 7
以下是一个简单的Android应用程序,使用Fragment实现了两个界面的切换:
MainActivity.java
```
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 加载Fragment1
Fragment1 fragment1 = new Fragment1();
getSupportFragmentManager().beginTransaction().add(R.id.fragment_container, fragment1).commit();
}
// 切换到Fragment2
public void switchToFragment2() {
Fragment2 fragment2 = new Fragment2();
getSupportFragmentManager().beginTransaction().replace(R.id.fragment_container, fragment2).commit();
}
// 切换回Fragment1
public void switchToFragment1() {
Fragment1 fragment1 = new Fragment1();
getSupportFragmentManager().beginTransaction().replace(R.id.fragment_container, fragment1).commit();
}
}
```
activity_main.xml
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.fragmentdemo.MainActivity">
<FrameLayout
android:id="@+id/fragment_container"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
```
Fragment1.java
```
public class Fragment1 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment1, container, false);
Button button = (Button) view.findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
((MainActivity) getActivity()).switchToFragment2();
}
});
return view;
}
}
```
fragment1.xml
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Switch to Fragment 2"
android:layout_centerInParent="true" />
</RelativeLayout>
```
Fragment2.java
```
public class Fragment2 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment2, container, false);
Button button = (Button) view.findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
((MainActivity) getActivity()).switchToFragment1();
}
});
return view;
}
}
```
fragment2.xml
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Switch to Fragment 1"
android:layout_centerInParent="true" />
</RelativeLayout>
```
这个应用程序包含两个Fragment,分别是Fragment1和Fragment2。在MainActivity中,我们使用FrameLayout作为容器,用来存放Fragment。在onCreate方法中,我们加载Fragment1。当点击Fragment1中的按钮时,会调用MainActivity中的switchToFragment2方法,用来切换到Fragment2。同样,当点击Fragment2中的按钮时,会调用MainActivity中的switchToFragment1方法,用来切换回Fragment1。
相关推荐
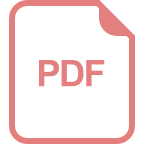














