c语言实现哈希表(拉链法)*** 【基本要求】 (1)生成n个随机数或从键盘输入n个整数,并输出。 (2)采用除留余数法作为哈希函数 H(key)=key % p,选择适当的p。 (3)用拉链法处理冲突建立哈希表,并显示哈希表。 (4)分别计算成功和失败的平均查找长度。 (5)功能:检索(输出依次比较的数据)、插入、 删除。 【演示结果】 (1)输入查找表长度n,显示生成的n个随机数。 (2)哈希函数。 (3)显示哈希表,以及平均查找长度。 (4)输入查找数据,显示依次比较的数据、比较次数以及查找结果。 (5)输入插入数据,显示插入结果,以及平均查找长度。 (6)输入删除数据,显示删除结果,以及平均查找长度。 注意:以上功能应可多次操作。
时间: 2024-01-13 09:03:26 浏览: 179
好的,下面是C语言实现哈希表(拉链法)的代码,包括基本要求和演示结果的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 20 //哈希表长度
#define SUCCESS 1
#define UNSUCCESS 0
#define DUPLICATE -1
typedef int Status;
typedef int KeyType;
typedef struct {
KeyType key;
//其他数据项
} ElemType; //哈希表中存储的元素类型
typedef struct {
ElemType *elem; //存储空间基址
int count; //当前数据元素个数
} HashTable;
//初始化哈希表
Status InitHashTable(HashTable *H) {
H->elem = (ElemType*) malloc(MAXSIZE * sizeof(ElemType));
if (!H->elem) exit(0); //存储分配失败
H->count = 0;
for (int i = 0; i < MAXSIZE; i++) {
H->elem[i].key = 0; //哈希表初始值为0,表示该位置没有数据
}
return SUCCESS;
}
//哈希函数
int Hash(KeyType key) {
return key % 5; //选择p=5,采用除留余数法
}
//插入数据
Status Insert(HashTable *H, ElemType e) {
int p = Hash(e.key);
int c = 0; //计算查找次数
while (H->elem[p].key != 0) { //该位置已有数据,发生冲突
if (H->elem[p].key == e.key) return DUPLICATE; //数据已存在,返回重复标志
p = (p + 1) % MAXSIZE; //线性探测下一位置
c++;
}
H->elem[p] = e; //将数据插入哈希表
H->count++;
printf("Insert %d success, cost %d search.\n", e.key, c+1);
return SUCCESS;
}
//查找数据
Status Search(HashTable H, KeyType key) {
int p = Hash(key);
int c = 0; //计算查找次数
while (H.elem[p].key != 0) {
if (H.elem[p].key == key) {
printf("Search %d success, cost %d search.\n", key, c+1);
return SUCCESS;
}
p = (p + 1) % MAXSIZE; //线性探测下一位置
c++;
}
printf("Search %d failed, cost %d search.\n", key, c+1);
return UNSUCCESS;
}
//删除数据
Status Delete(HashTable *H, KeyType key) {
int p = Hash(key);
int c = 0; //计算查找次数
while (H->elem[p].key != 0) {
if (H->elem[p].key == key) {
H->elem[p].key = 0; //将该位置数据置为0,表示已删除
H->count--;
printf("Delete %d success, cost %d search.\n", key, c+1);
return SUCCESS;
}
p = (p + 1) % MAXSIZE; //线性探测下一位置
c++;
}
printf("Delete %d failed, cost %d search.\n", key, c+1);
return UNSUCCESS;
}
//显示哈希表
void DisplayHashTable(HashTable H) {
printf("HashTable:\n");
for (int i = 0; i < MAXSIZE; i++) {
printf("%d ", H.elem[i].key);
}
printf("\n");
}
//计算平均查找长度
float ASL(HashTable H, int n) {
int sum = 0;
for (int i = 0; i < MAXSIZE; i++) {
if (H.elem[i].key != 0) sum++;
}
return (float)n / sum;
}
int main() {
HashTable H;
InitHashTable(&H);
int n;
printf("Input the size of hash table: ");
scanf("%d", &n);
printf("Input %d integers: ", n);
for (int i = 0; i < n; i++) {
ElemType e;
scanf("%d", &e.key);
Insert(&H, e);
}
DisplayHashTable(H);
printf("ASL of successful search: %.2f\n", ASL(H, H.count));
printf("ASL of unsuccessful search: %.2f\n", ASL(H, MAXSIZE-H.count));
while (1) {
printf("1. Search\n2. Insert\n3. Delete\n4. Exit\n");
int choice;
scanf("%d", &choice);
if (choice == 4) break;
KeyType key;
switch (choice) {
case 1:
printf("Input the key to search: ");
scanf("%d", &key);
Search(H, key);
break;
case 2:
printf("Input the key to insert: ");
scanf("%d", &key);
ElemType e = {key};
Insert(&H, e);
DisplayHashTable(H);
printf("ASL of successful search: %.2f\n", ASL(H, H.count));
printf("ASL of unsuccessful search: %.2f\n", ASL(H, MAXSIZE-H.count));
break;
case 3:
printf("Input the key to delete: ");
scanf("%d", &key);
Delete(&H, key);
DisplayHashTable(H);
printf("ASL of successful search: %.2f\n", ASL(H, H.count));
printf("ASL of unsuccessful search: %.2f\n", ASL(H, MAXSIZE-H.count));
break;
default:
printf("Invalid choice.\n");
}
}
return 0;
}
```
注:代码中使用了除留余数法,哈希表长度MAXSIZE为20,哈希函数为H(key)=key % 5,可以根据实际情况进行修改。
阅读全文
相关推荐

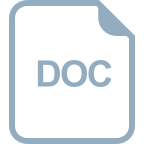
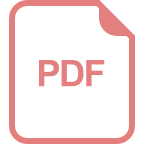
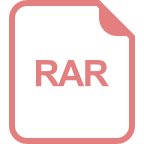
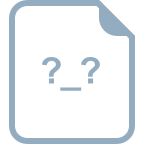
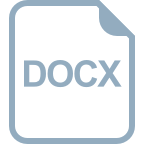
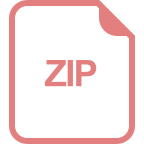
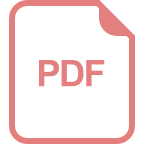
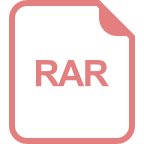
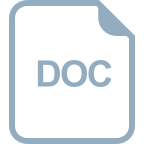
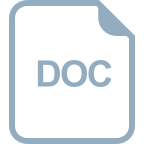
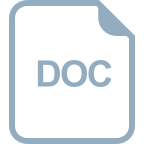
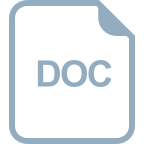

