c++学生信息管理系统,学生信息包括学号,姓名,年龄,性别,班级等信息;小学生除了包括学生所有信息外还包括语文数学英语成绩;中学生除了包括小学生所有信息外还包括地理历史成绩和家庭住址等信息;大学生除了包括学生所有信息外还包括专业,家庭住址,联系方式等信息
时间: 2024-04-17 17:29:15 浏览: 26
您可以通过创建一个基类学生信息类(Student)来存储所有学生共有的信息(学号、姓名、年龄、性别、班级),然后创建派生类小学生信息类(PrimaryStudent)、中学生信息类(MiddleStudent)和大学生信息类(CollegeStudent)来存储各自特有的信息。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 基类:学生信息
class Student {
protected:
int studentID;
string name;
int age;
string gender;
string className;
public:
// 构造函数
Student(int id, string n, int a, string g, string c)
: studentID(id), name(n), age(a), gender(g), className(c) {}
// 显示学生信息
void displayInfo() {
cout << "学号:" << studentID << endl;
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "性别:" << gender << endl;
cout << "班级:" << className << endl;
}
};
// 派生类:小学生信息
class PrimaryStudent : public Student {
private:
int chineseScore;
int mathScore;
int englishScore;
public:
// 构造函数
PrimaryStudent(int id, string n, int a, string g, string c, int chinese, int math, int english)
: Student(id, n, a, g, c), chineseScore(chinese), mathScore(math), englishScore(english) {}
// 显示小学生信息
void displayInfo() {
Student::displayInfo();
cout << "语文成绩:" << chineseScore << endl;
cout << "数学成绩:" << mathScore << endl;
cout << "英语成绩:" << englishScore << endl;
}
};
// 派生类:中学生信息
class MiddleStudent : public PrimaryStudent {
private:
int geographyScore;
int historyScore;
string homeAddress;
public:
// 构造函数
MiddleStudent(int id, string n, int a, string g, string c, int chinese, int math, int english,
int geography, int history, string address)
: PrimaryStudent(id, n, a, g, c), geographyScore(geography), historyScore(history), homeAddress(address) {}
// 显示中学生信息
void displayInfo() {
PrimaryStudent::displayInfo();
cout << "地理成绩:" << geographyScore << endl;
cout << "历史成绩:" << historyScore << endl;
cout << "家庭住址:" << homeAddress << endl;
}
};
// 派生类:大学生信息
class CollegeStudent : public Student {
private:
string major;
string homeAddress;
string contact;
public:
// 构造函数
CollegeStudent(int id, string n, int a, string g, string c, string m, string address, string ct)
: Student(id, n, a, g, c), major(m), homeAddress(address), contact(ct) {}
// 显示大学生信息
void displayInfo() {
Student::displayInfo();
cout << "专业:" << major << endl;
cout << "家庭住址:" << homeAddress << endl;
cout << "联系方式:" << contact << endl;
}
};
int main() {
// 创建一个小学生对象
PrimaryStudent primaryStu(1001, "小明", 10, "男", "一年级", 90, 80, 95);
cout << "小学生信息:" << endl;
primaryStu.displayInfo();
cout << endl;
// 创建一个中学生对象
MiddleStudent middleStu(2001, "小红", 15, "女", "初一", 85, 90, 80, 75, 80, "上海市");
cout << "中学生信息:" << endl;
middleStu.displayInfo();
cout << endl;
// 创建一个大学生对象
CollegeStudent collegeStu(3001, "小李", 20, "男", "计算机科学与技术", "北京市", "18888888888");
cout << "大学生信息:" << endl;
collegeStu.displayInfo();
cout << endl;
return 0;
}
```
这个示例代码中定义了一个基类 `Student`,包含了学号、姓名、年龄、性别和班级等信息。然后通过继承的方式创建了派生类 `PrimaryStudent`(小学生)、`MiddleStudent`(中学生)和 `CollegeStudent`(大学生)来添加各自特有的信息。
您可以根据需求进一步扩展这些类,比如添加成绩计算、信息修改等功能。希望对您有帮助!
相关推荐
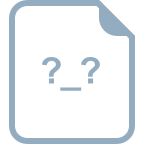
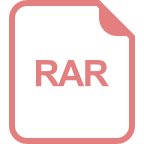
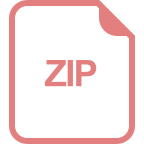













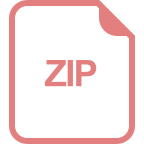
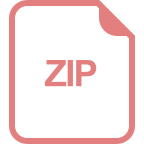