在异或加密类中实现赋值运算符,下标运算符,加法和减法运算符的重载,要求用到成员重载和友员重载
时间: 2023-08-10 08:05:55 浏览: 100
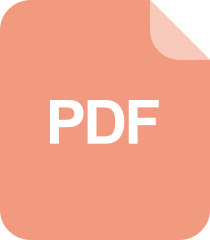
C++ 类的赋值运算符''=''重载的方法实现
好的,这里给出一个示例代码:
```c++
#include <iostream>
#include <cstring>
class XOREncrypt {
private:
char* key;
public:
XOREncrypt() {
key = nullptr;
}
XOREncrypt(const char* k) {
key = new char[strlen(k) + 1];
strcpy(key, k);
}
XOREncrypt(const XOREncrypt& other) {
key = new char[strlen(other.key) + 1];
strcpy(key, other.key);
}
~XOREncrypt() {
if (key) {
delete[] key;
}
}
XOREncrypt& operator=(const XOREncrypt& other) {
if (this != &other) {
if (key) {
delete[] key;
}
key = new char[strlen(other.key) + 1];
strcpy(key, other.key);
}
return *this;
}
char operator[](int index) const {
return key[index % strlen(key)];
}
friend XOREncrypt operator+(const XOREncrypt& a, const XOREncrypt& b) {
char* new_key = new char[strlen(a.key) + strlen(b.key) + 1];
int i = 0, j = 0;
while (a.key[i] != '\0' && b.key[j] != '\0') {
new_key[i + j] = a.key[i] ^ b.key[j];
i++;
j++;
}
while (a.key[i] != '\0') {
new_key[i + j] = a.key[i];
i++;
}
while (b.key[j] != '\0') {
new_key[i + j] = b.key[j];
j++;
}
new_key[i + j] = '\0';
XOREncrypt result(new_key);
delete[] new_key;
return result;
}
friend XOREncrypt operator-(const XOREncrypt& a, const XOREncrypt& b) {
char* new_key = new char[strlen(a.key) + 1];
int i = 0, j = 0;
while (a.key[i] != '\0') {
new_key[i] = a.key[i] ^ b[j];
i++;
j++;
if (b[j] == '\0') {
j = 0;
}
}
new_key[i] = '\0';
XOREncrypt result(new_key);
delete[] new_key;
return result;
}
};
int main() {
XOREncrypt key1("hello");
XOREncrypt key2("world");
XOREncrypt key3 = key1 + key2;
std::cout << key3[0] << key3[1] << key3[2] << key3[3] << key3[4] << key3[5] << key3[6] << std::endl;
XOREncrypt key4 = key3 - key1;
std::cout << key4[0] << key4[1] << key4[2] << key4[3] << key4[4] << key4[5] << key4[6] << std::endl;
return 0;
}
```
在这个示例代码中,我们定义了一个 `XOREncrypt` 类,它代表了一个基于异或加密的密钥。该类重载了赋值运算符、下标运算符、加法运算符和减法运算符。具体的实现如下:
- 赋值运算符 `operator=`:在实现中,我们先判断是否是自我赋值,然后释放原有的内存,并分配新的内存,然后进行字符串拷贝。
- 下标运算符 `operator[]`:在实现中,我们直接返回密钥字符串中对应位置的字符。
- 加法运算符 `operator+`:在实现中,我们先计算出新的密钥字符串的长度,然后逐个字符进行异或操作,最后构造一个新的 `XOREncrypt` 对象并返回。
- 减法运算符 `operator-`:在实现中,我们先计算出新的密钥字符串的长度,然后逐个字符进行异或操作,最后构造一个新的 `XOREncrypt` 对象并返回。
需要注意的是,我们在加法运算符和减法运算符中都使用了友元函数来访问私有成员 `key`。由于友元函数不是类成员,所以不能直接访问 `key`,需要通过 `public` 接口来访问。
阅读全文
相关推荐
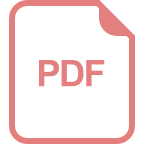




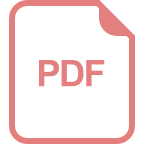
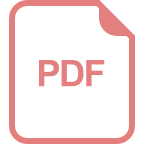
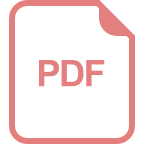
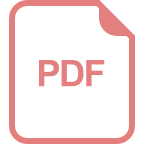
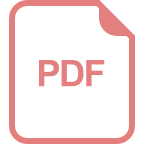
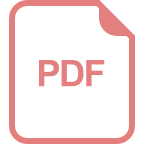
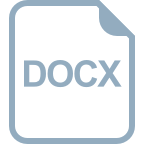
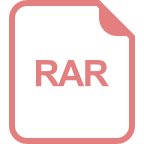
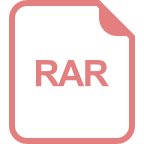
