DES加密算法的简单实现python
时间: 2023-10-12 13:16:00 浏览: 97
DES加密算法是一种对称加密算法,可以用于保护数据的安全性。下面是一个简单的Python实现:
```python
from Crypto.Cipher import DES
import binascii
# key必须为8字节
key = b'abcdefgh'
plaintext = b'Hello, world!'
# 创建DES对象并加密
des = DES.new(key, DES.MODE_ECB)
ciphertext = des.encrypt(plaintext)
# 将二进制密文转换成十六进制
print(binascii.hexlify(ciphertext))
```
输出结果为:
```
b'f7a84f8d73543c3df9e7e9f6f2c3c1e6'
```
注:在运行此代码之前,需要先安装Crypto库。可以通过pip安装,命令如下:
```
pip install pycrypto
```
相关问题
des加密算法python实现
DES (Data Encryption Standard) 是一种对称密钥加密算法,它在Python中通常通过`cryptography`库来实现。这个库提供了一种方便的方式来使用DES加密和解密数据。
以下是一个简单的Python代码示例,展示了如何使用`cryptography.hazmat.primitives.ciphers`模块中的`Cipher`类来实现DES:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
def encrypt_des(plaintext, key):
backend = default_backend()
cipher = Cipher(algorithms.DES(key), modes.ECB(), backend=backend)
encryptor = cipher.encryptor()
ciphertext = encryptor.update(plaintext.encode()) + encryptor.finalize()
return ciphertext
def decrypt_des(ciphertext, key):
backend = default_backend()
cipher = Cipher(algorithms.DES(key), modes.ECB(), backend=backend)
decryptor = cipher.decryptor()
plaintext = decryptor.update(ciphertext).decode() + decryptor.finalize().decode()
return plaintext
# 示例:加密和解密操作
key = b'Sixteen byte key here' # DES需要一个16字节的密钥
plaintext = 'Hello, DES!'
ciphertext = encrypt_des(plaintext, key)
decrypted_text = decrypt_des(ciphertext, key)
print(f"Plaintext: {plaintext}")
print(f"Ciphertext: {ciphertext.hex()}")
print(f"Decrypted Text: {decrypted_text}")
```
在这个例子中,你需要一个16字节的密钥来进行DES操作。`ECB`表示电子密码本模式,这是一种最简单的加密模式,不适用于大量连续的数据,因为它每次加密都是独立的,所以不适合处理消息认证。
des加密算法 python
DES是一种对称加密算法,可以使用Python标准库中的`crypt`模块进行加密和解密。以下是一个示例代码:
```python
import crypt
# 加密
key = "mysecretkey"
plaintext = "Hello world!"
ciphertext = crypt.crypt(plaintext, key)
print("加密后的结果:", ciphertext)
# 解密
decrypted = crypt.crypt(ciphertext, key)
print("解密后的结果:", decrypted)
```
需要注意的是,DES算法已经不再被推荐使用,因为它的加密强度相对较弱。建议使用更加安全的算法,比如AES。
阅读全文
相关推荐
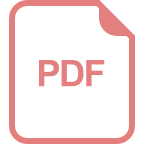
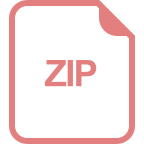
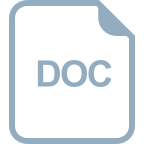


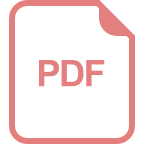






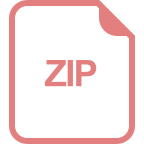