MySQL 如何将从一个字符串中清除调另一个字符串中的值
时间: 2024-03-10 09:50:12 浏览: 20
可以使用MySQL的字符串函数 `REPLACE` 和 `TRIM` 来清除一个字符串中另一个字符串中的值。
假设有一个表 `test`,其中有一列 `string1` 存储了一个字符串,另一列 `string2` 存储了需要清除的值。现在要清除 `string1` 中 `string2` 中包含的值。可以使用以下SQL语句实现:
```
SELECT TRIM(BOTH ',' FROM REPLACE(CONCAT(',', string1, ','), CONCAT(',', string2, ','), ',')) AS cleaned_string
FROM test;
```
以上 SQL 语句中,使用 `CONCAT` 函数将 `string1` 前后都添加上逗号,以便于后续的处理。然后使用 `REPLACE` 函数将 `string1` 中包含的 `string2` 替换为空字符串。最后使用 `TRIM` 函数去除字符串前后的逗号。
例如,对于 `string1 = "1,2,3,4,5,6,7,8,9"`,`string2 = "3,5,7"`,使用以上 SQL 语句可以得到以下结果:
```
cleaned_string
---------------
1,2,4,6,8,9
```
这样就可以将 `string1` 中 `string2` 中包含的值清除掉,并将清除后的结果存储到一个新的表或者将其更新到原表中。
相关问题
用java代码实现读取一个文件中所以的txt文件逐行读取txt文件中的内容清除所以空格和换行,判断如果等于字符串[INTRO]时,之后的读取内容方式改为两行读取内容,内容不能为空格和换行,如果等于字符串优待政策时,就改为多行读取,结束标记为服务设施,如果等于字符串服务设施时,就改为多行读取,结束标记为[GRADE],如果等于字符串[GRADE]时,就按照逐行读取内容,最后将这些数据放入数据库中
以下Java代码实现读取文件中的内容,并根据不同的标记进行不同的读取方式和处理:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class ReadFile {
public static void main(String[] args) {
String filePath = "file.txt"; // 文件路径
String line;
String introContent = ""; // [INTRO]标记后的内容
String policyContent = ""; // [优待政策]标记后的内容
String facilityContent = ""; // [服务设施]标记后的内容
String gradeContent = ""; // [GRADE]标记后的内容
boolean isIntro = false; // 是否已经读取到[INTRO]
boolean isPolicy = false; // 是否已经读取到[优待政策]
boolean isFacility = false; // 是否已经读取到[服务设施]
boolean isGrade = false; // 是否已经读取到[GRADE]
boolean isContent = false; // 是否正在读取内容
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
while ((line = br.readLine()) != null) {
// 清除空格和换行
line = line.replaceAll("\\s", "");
if (line.equals("[INTRO]")) {
isIntro = true;
isContent = false;
} else if (line.equals("优待政策")) {
isPolicy = true;
isContent = false;
} else if (line.equals("服务设施")) {
isFacility = true;
isContent = false;
} else if (line.equals("[GRADE]")) {
isGrade = true;
isContent = false;
} else if (!line.isEmpty()) { // 如果不是空行
if (isIntro) { // [INTRO]后的内容
if (!isContent) {
introContent += line; // 读取第一行
isContent = true;
} else {
introContent += line; // 读取第二行
isIntro = false;
}
} else if (isPolicy || isFacility || isGrade) { // 多行读取
if (!line.equals("服务设施") && !line.equals("[GRADE]")) {
if (!isContent) {
isContent = true;
} else {
policyContent += "\n";
facilityContent += "\n";
gradeContent += "\n";
}
policyContent += line;
facilityContent += line;
gradeContent += line;
} else {
isPolicy = false;
isFacility = false;
isGrade = false;
isContent = false;
}
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
// 将数据存入数据库
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
PreparedStatement ps = conn.prepareStatement("INSERT INTO test_data (intro, policy, facility, grade) VALUES (?, ?, ?, ?)");) {
ps.setString(1, introContent);
ps.setString(2, policyContent);
ps.setString(3, facilityContent);
ps.setString(4, gradeContent);
ps.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
需要注意的是,这段代码中的数据库连接和表名等需要根据实际情况进行修改。
用jsp+sql写一个简单的图书管理系统
好的,我可以给您提供一个简单的图书管理系统的参考实现,使用JSP和SQL实现。
首先,数据库需要创建一个名为“books”的表,包含以下字段:
- id: 图书ID,自增长整数
- name: 图书名称,字符串
- author: 作者,字符串
- publisher: 出版社,字符串
- publishDate: 出版日期,日期类型
- price: 价格,浮点数类型
接下来,我们可以创建以下JSP页面来实现图书管理系统:
1. index.jsp:系统首页,包含“添加图书”、“查看图书”和“退出系统”三个链接。
```jsp
<html>
<head>
<title>图书管理系统</title>
</head>
<body>
<h1>图书管理系统</h1>
<a href="add.jsp">添加图书</a><br>
<a href="list.jsp">查看图书</a><br>
<a href="logout.jsp">退出系统</a>
</body>
</html>
```
2. add.jsp:添加图书页面,包含一个表单,可以输入图书信息。
```jsp
<html>
<head>
<title>添加图书</title>
</head>
<body>
<h1>添加图书</h1>
<form action="add.do" method="post">
<label>图书名称:</label><input type="text" name="name"><br>
<label>作者:</label><input type="text" name="author"><br>
<label>出版社:</label><input type="text" name="publisher"><br>
<label>出版日期:</label><input type="text" name="publishDate"><br>
<label>价格:</label><input type="text" name="price"><br>
<input type="submit" value="添加">
</form>
</body>
</html>
```
3. add.do:添加图书处理页面,将表单提交到此页面,将表单数据插入到数据库中,并跳转到“查看图书”页面。
```jsp
<%@ page import="java.sql.*" %>
<%
String name = request.getParameter("name");
String author = request.getParameter("author");
String publisher = request.getParameter("publisher");
String publishDate = request.getParameter("publishDate");
String price = request.getParameter("price");
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "123456");
String sql = "insert into books(name, author, publisher, publishDate, price) values (?, ?, ?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, name);
stmt.setString(2, author);
stmt.setString(3, publisher);
stmt.setString(4, publishDate);
stmt.setFloat(5, Float.parseFloat(price));
stmt.executeUpdate();
response.sendRedirect("list.jsp");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (Exception e) {}
}
%>
```
4. list.jsp:查看图书页面,从数据库中查询所有图书信息,并显示在表格中。
```jsp
<%@ page import="java.sql.*" %>
<html>
<head>
<title>查看图书</title>
</head>
<body>
<h1>查看图书</h1>
<table border="1">
<tr>
<th>ID</th>
<th>图书名称</th>
<th>作者</th>
<th>出版社</th>
<th>出版日期</th>
<th>价格</th>
</tr>
<%
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "123456");
String sql = "select * from books";
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
Date publishDate = rs.getDate("publishDate");
float price = rs.getFloat("price");
%>
<tr>
<td><%= id %></td>
<td><%= name %></td>
<td><%= author %></td>
<td><%= publisher %></td>
<td><%= publishDate %></td>
<td><%= price %></td>
</tr>
<%
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (Exception e) {}
}
%>
</table>
</body>
</html>
```
5. logout.jsp:退出系统页面,清除session,并跳转到系统首页。
```jsp
<%
session.invalidate();
response.sendRedirect("index.jsp");
%>
```
以上就是一个简单的图书管理系统的JSP和SQL实现,供您参考。
相关推荐
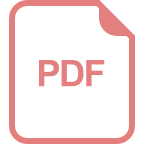
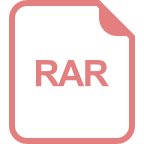







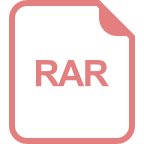
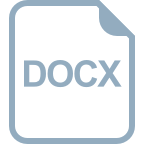
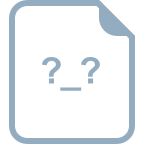
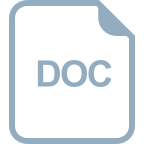
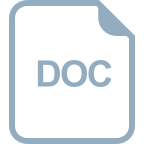
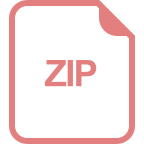
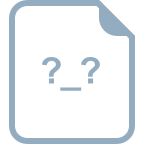