如何利用tkinter弹出一个窗口
时间: 2023-12-20 12:50:11 浏览: 60
您可以使用以下代码在Python中使用tkinter弹出一个窗口:
```python
import tkinter as tk
# 创建主窗口
root = tk.Tk()
# 添加标签
label = tk.Label(root, text="Hello World!")
label.pack()
# 运行主循环
root.mainloop()
```
这将创建一个带有“Hello World!”文本的窗口。
相关问题
tkinter弹出另一个窗口
要弹出另一个窗口,您需要使用Tkinter的Toplevel小部件。以下是一个简单的示例:
```python
import tkinter as tk
class AnotherWindow(tk.Toplevel):
def __init__(self, parent):
super().__init__(parent)
self.title('Another Window')
self.geometry('200x200')
self.label = tk.Label(self, text='This is another window')
self.label.pack(padx=20, pady=20)
class MainWindow(tk.Tk):
def __init__(self):
super().__init__()
self.title('Main Window')
self.geometry('200x200')
self.button = tk.Button(self, text='Open Another Window', command=self.open_window)
self.button.pack(padx=20, pady=20)
def open_window(self):
another_window = AnotherWindow(self)
if __name__ == '__main__':
app = MainWindow()
app.mainloop()
```
在此示例中,我们定义了两个类:MainWindow和AnotherWindow。 MainWindow是我们的主窗口,其中包含一个按钮,用于打开AnotherWindow。 AnotherWindow是我们要弹出的第二个窗口。
在AnotherWindow构造函数中,我们使用Toplevel小部件创建一个新窗口。我们还创建了一个标签小部件并将其添加到窗口中。
在MainWindow中,我们将按钮添加到窗口中,并将其命令设置为open_window方法。该方法创建AnotherWindow实例并将其传递给self(MainWindow)作为其父窗口。
当我们单击按钮时,open_window方法将创建AnotherWindow实例并将其显示在屏幕上。
tkinter弹出两个窗口如何解决
可以采用以下两种方法来解决tkinter弹出两个窗口的问题:
1.使用Toplevel()函数创建一个子窗口,而不是使用Tk()函数创建一个新的主窗口。这样,你就不会再创建一个新的主窗口了。例如:
```python
import tkinter as tk
root = tk.Tk()
root.title("Main Window")
def open_window():
# 创建一个子窗口
top = tk.Toplevel()
top.title("Sub Window")
btn = tk.Button(root, text="Open Window", command=open_window)
btn.pack()
root.mainloop()
```
2.使用withdraw()方法和deiconify()方法来隐藏和显示主窗口。当你点击“Open Window”按钮时,先使用withdraw()方法隐藏主窗口,然后创建一个新的子窗口,并在子窗口关闭后再使用deiconify()方法显示主窗口。例如:
```python
import tkinter as tk
root = tk.Tk()
root.title("Main Window")
def open_window():
# 隐藏主窗口
root.withdraw()
# 创建一个子窗口
top = tk.Toplevel()
top.title("Sub Window")
# 子窗口关闭时再显示主窗口
top.protocol("WM_DELETE_WINDOW", lambda: root.deiconify())
btn = tk.Button(root, text="Open Window", command=open_window)
btn.pack()
root.mainloop()
```
这两种方法都可以解决弹出两个窗口的问题,选择哪种方法取决于你的具体需求。
阅读全文
相关推荐
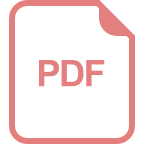
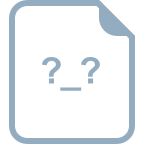
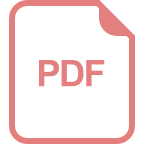












