用C语言设计一个外卖点餐系统
时间: 2024-02-25 19:59:39 浏览: 28
好的,设计一个外卖点餐系统可以分成以下几个部分:
1. 用户登录:用户需要输入用户名和密码才能登录系统。如果是新用户,需要进行注册。
2. 菜单展示:系统需要展示当前可供选择的菜单,包括菜名、价格等信息。
3. 点餐功能:用户可以选择自己喜欢的菜品,并选择数量。
4. 购物车:系统需要记录用户已经选择的菜品和数量,计算总价。
5. 结算:用户可以选择支付方式,系统需要进行支付操作并生成订单。
6. 订单管理:系统需要记录用户的订单信息,并提供查询、修改、删除等功能。
在C语言中设计外卖点餐系统的话,可以使用结构体来存储菜单、购物车、订单等信息。
以下是代码示例:
```
#include <stdio.h>
#include <string.h>
struct food {
char name[20];
float price;
};
struct cart {
struct food item;
int quantity;
};
struct order {
struct cart cart_items[10];
float total_price;
char payment_method[20];
};
int main() {
struct food menu[5] = {{"Pizza", 9.99}, {"Burger", 6.99}, {"Fried Chicken", 8.99}, {"Salad", 5.99}, {"Sushi", 12.99}};
struct cart my_cart[10];
struct order my_order;
int menu_choice, quantity, cart_index = 0;
float total_price = 0;
char username[20], password[20], payment_method[20];
// 用户登录
printf("Enter your username: ");
scanf("%s", username);
printf("Enter your password: ");
scanf("%s", password);
// 菜单展示
printf("Welcome to our restaurant! Here is our menu:\n");
for (int i = 0; i < 5; i++) {
printf("%d. %s ($%.2f)\n", i+1, menu[i].name, menu[i].price);
}
// 点餐功能
printf("Enter the menu item number you want to order: ");
scanf("%d", &menu_choice);
printf("Enter the quantity you want to order: ");
scanf("%d", &quantity);
// 添加到购物车
my_cart[cart_index].item = menu[menu_choice-1];
my_cart[cart_index].quantity = quantity;
cart_index++;
total_price += menu[menu_choice-1].price * quantity;
// 购物车展示
printf("Your cart:\n");
for (int i = 0; i < cart_index; i++) {
printf("%d. %s x %d ($%.2f)\n", i+1, my_cart[i].item.name, my_cart[i].quantity, my_cart[i].item.price * my_cart[i].quantity);
}
printf("Total price: $%.2f\n", total_price);
// 结算
printf("Enter your payment method: ");
scanf("%s", payment_method);
strcpy(my_order.payment_method, payment_method);
my_order.total_price = total_price;
// 订单管理
printf("Your order has been placed! Here are your order details:\n");
for (int i = 0; i < cart_index; i++) {
printf("%d. %s x %d ($%.2f)\n", i+1, my_cart[i].item.name, my_cart[i].quantity, my_cart[i].item.price * my_cart[i].quantity);
}
printf("Total price: $%.2f\n", total_price);
printf("Payment method: %s\n", my_order.payment_method);
return 0;
}
```
相关推荐
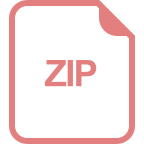
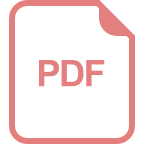
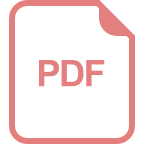












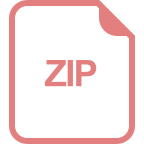
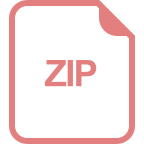