帮我写一个lzw编码程序
时间: 2023-10-03 16:04:43 浏览: 45
好的,下面是 Python 代码实现 LZW 编码:
```python
def lzw_encode(text):
# 初始化编码字典,将 ASCII 0~255 的字符作为键,值为对应的编码
dictionary = {chr(i): i for i in range(256)}
result = [] # 存放编码结果的列表
w = "" # 当前匹配字符串
for c in text:
wc = w + c
if wc in dictionary: # 如果当前匹配字符串在字典中存在
w = wc # 将当前匹配字符串更新为 wc
else:
result.append(dictionary[w])
dictionary[wc] = len(dictionary) # 将 wc 添加到字典中
w = c # 将当前匹配字符串更新为 c
if w:
result.append(dictionary[w])
return result
```
其中 `text` 是需要编码的字符串,返回值是一个列表,存放编码后的结果。下面是一个例子:
```python
text = "ABABABA"
encoded = lzw_encode(text)
print(encoded) # [65, 66, 256, 258, 260]
```
如果需要将编码后的结果转换成二进制字符串,可以使用以下代码:
```python
def to_binary_string(encoded, num_bits):
# 将编码结果转换为固定位数的二进制字符串
return "".join(format(code, "0" + str(num_bits) + "b") for code in encoded)
# 将编码结果转换为 12 位的二进制字符串
binary_str = to_binary_string(encoded, 12)
print(binary_str) # 010000010010000101000000100000010100000101000001
```
这里的 `num_bits` 是指每个编码需要的二进制位数,这里设为 12。`format(code, "0" + str(num_bits) + "b")` 表示将 `code` 转换为 12 位的二进制字符串,不足 12 位的前面补零。
相关推荐
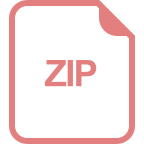














