代码实现 C语言 解析xxx.xxx.xxx字符串 分别解析出xxx xxx xxx
时间: 2023-09-29 21:03:09 浏览: 289
解析xxx.xxx.xxx字符串的C语言代码如下:
```
#include <stdio.h>
#include <string.h>
int main()
{
char str[] = "xxx.xxx.xxx";
char *token = strtok(str, ".");
while (token != NULL)
{
printf("%s ", token);
token = strtok(NULL, ".");
}
return 0;
}
```
以上代码可以分别解析出字符串中的 xxx, xxx, xxx 三段内容。
相关问题
用字符串表示的IP地址的格式是“xxx.xxx.xxx.xxx”,但IP地址在内存中是一个unsigned int,字符串格式就是将这个unsigned int的4个字节unsigned char分别转换成字符串,然后中间用小数点隔开。编写程序,使用指针技术,将一个unsigned int以字符串IP地址的格式打印出来。
以下是一个使用指针技术将unsigned int转换为字符串IP地址格式的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void int_to_ip(unsigned int ip, char *buffer) {
unsigned char bytes[4];
char *ptr = buffer;
memcpy(bytes, &ip, sizeof(unsigned int));
for (int i = 0; i < 4; i++) {
sprintf(ptr, "%d", bytes[i]);
ptr += strlen(ptr);
if (i < 3) {
*ptr++ = '.';
}
}
}
int main() {
unsigned int ip = 0xC0A80001; // IP地址 192.168.0.1
char buffer[16]; // IP地址字符串的缓冲区
int_to_ip(ip, buffer);
printf("%s\n", buffer); // 输出 "192.168.0.1"
return 0;
}
```
这个程序定义了一个`int_to_ip`函数,它接受一个unsigned int格式的IP地址和一个字符串缓冲区,然后将这个IP地址转换为字符串IP地址格式并存储在缓冲区中。这个函数使用指针技术来处理字符串缓冲区,并且使用sprintf函数将每个字节转换为十进制字符串,并在每两个字节之间插入一个小数点。
【问题描述】用C语言编写一个程序,提示用户以(xxx)xxx-xxxx的格式输入电话号码,并以xxx.xxx.xxxx的格式显示该号码。
在C语言中,你可以通过使用`scanf()`函数获取用户的输入,并使用字符串操作函数如`strcpy()`、`strcat()`等来处理和转换电话号码的格式。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
int main() {
char phone_input[20];
char output[15];
// 提示用户输入电话号码
printf("请输入电话号码 (xxx)xxx-xxxx 的格式: ");
scanf("%s", phone_input); // 输入格式控制符匹配括号内的格式
// 分割并格式化电话号码
int i = 0;
while (phone_input[i] != '\0') { // 遍历输入字符串
if (phone_input[i] == '(' && phone_input[i + 1] == 'x') { // 找到第一个空格位置
phone_input[i] = '\0'; // 结束前面部分
strcat(output, "XXX."); // 输出前三位加句点
i += 4; // 跳过四位数字和空格
} else if (isdigit(phone_input[i])) { // 如果是数字
output[strlen(output)] = phone_input[i]; // 添加到输出字符串
}
i++;
}
// 结尾添加四位数字
strcat(output, "XXXX");
printf("显示的电话号码格式: %s\n", output);
return 0;
}
阅读全文
相关推荐
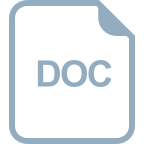
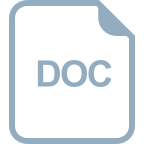
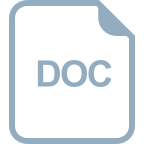
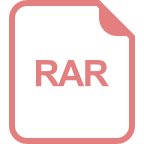
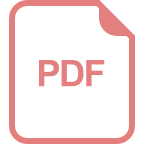
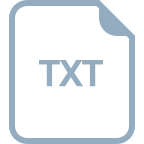
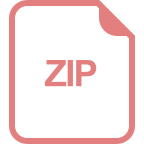







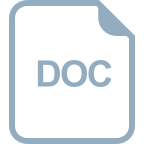
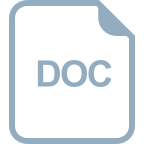
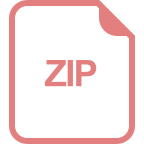