利用线性链表实现学生通信录管理系统,具体功能:输入、输出、插入、删除、查找、追加、读入、显示、拷贝、排序、退出,并能在屏幕上输出操作前后的结果。
时间: 2023-07-04 16:07:44 浏览: 97
好的,我可以为您介绍一下如何利用线性链表实现学生通信录管理系统。
首先,我们需要定义一个学生信息结构体,包含学号、姓名、电话等信息。然后定义一个链表节点结构体,包含学生信息和指向下一个节点的指针。接下来,我们可以定义一个链表结构体,包含头指针和尾指针,方便进行插入和删除操作。
具体实现如下:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
string phone;
};
struct Node {
Student data;
Node* next;
};
struct List {
Node* head;
Node* tail;
};
// 初始化链表
void initList(List& list) {
list.head = nullptr;
list.tail = nullptr;
}
// 插入节点
void insertNode(List& list, Node* node) {
if (list.head == nullptr) {
list.head = node;
list.tail = node;
}
else {
list.tail->next = node;
list.tail = node;
}
}
// 删除节点
void deleteNode(List& list, Node* node) {
if (node == list.head) {
list.head = node->next;
}
else {
Node* prev = list.head;
while (prev->next != node) {
prev = prev->next;
}
prev->next = node->next;
if (node == list.tail) {
list.tail = prev;
}
}
delete node;
}
// 查找节点
Node* findNode(List& list, int id) {
Node* node = list.head;
while (node != nullptr) {
if (node->data.id == id) {
return node;
}
node = node->next;
}
return nullptr;
}
// 追加节点
void appendNode(List& list, Node* node) {
if (list.head == nullptr) {
list.head = node;
list.tail = node;
}
else {
node->next = list.head;
list.head = node;
}
}
// 读入学生信息
Student readStudent() {
Student student;
cout << "请输入学号:";
cin >> student.id;
cout << "请输入姓名:";
cin >> student.name;
cout << "请输入电话:";
cin >> student.phone;
return student;
}
// 显示学生信息
void displayStudent(const Student& student) {
cout << "学号:" << student.id << endl;
cout << "姓名:" << student.name << endl;
cout << "电话:" << student.phone << endl;
}
// 显示链表所有节点信息
void displayList(const List& list) {
Node* node = list.head;
while (node != nullptr) {
displayStudent(node->data);
cout << endl;
node = node->next;
}
}
// 拷贝链表
void copyList(List& dest, const List& src) {
Node* node = src.head;
while (node != nullptr) {
Node* newNode = new Node;
newNode->data = node->data;
newNode->next = nullptr;
insertNode(dest, newNode);
node = node->next;
}
}
// 排序链表
void sortList(List& list) {
// 使用选择排序算法
Node* i = list.head;
while (i != nullptr) {
Node* j = i->next;
while (j != nullptr) {
if (i->data.id > j->data.id) {
swap(i->data, j->data);
}
j = j->next;
}
i = i->next;
}
}
// 主函数
int main() {
List list;
initList(list);
while (true) {
cout << "请选择操作:1-输入,2-输出,3-插入,4-删除,5-查找,6-追加,7-读入,8-显示,9-拷贝,10-排序,0-退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1: {
Student student = readStudent();
Node* node = new Node;
node->data = student;
node->next = nullptr;
insertNode(list, node);
cout << "插入成功!" << endl;
break;
}
case 2: {
displayList(list);
break;
}
case 3: {
cout << "请输入要插入的学生信息:" << endl;
Student student = readStudent();
Node* node = new Node;
node->data = student;
node->next = nullptr;
int id;
cout << "请输入要插入的位置的学号:" << endl;
cin >> id;
Node* prev = findNode(list, id);
if (prev == nullptr) {
cout << "未找到该学号的节点!" << endl;
}
else {
node->next = prev->next;
prev->next = node;
cout << "插入成功!" << endl;
}
break;
}
case 4: {
int id;
cout << "请输入要删除的学号:" << endl;
cin >> id;
Node* node = findNode(list, id);
if (node == nullptr) {
cout << "未找到该学号的节点!" << endl;
}
else {
deleteNode(list, node);
cout << "删除成功!" << endl;
}
break;
}
case 5: {
int id;
cout << "请输入要查找的学号:" << endl;
cin >> id;
Node* node = findNode(list, id);
if (node == nullptr) {
cout << "未找到该学号的节点!" << endl;
}
else {
displayStudent(node->data);
}
break;
}
case 6: {
Student student = readStudent();
Node* node = new Node;
node->data = student;
node->next = nullptr;
appendNode(list, node);
cout << "追加成功!" << endl;
break;
}
case 7: {
int n;
cout << "请输入要读入的学生数量:" << endl;
cin >> n;
for (int i = 0; i < n; i++) {
Student student = readStudent();
Node* node = new Node;
node->data = student;
node->next = nullptr;
insertNode(list, node);
}
cout << "读入成功!" << endl;
break;
}
case 8: {
displayList(list);
break;
}
case 9: {
List newList;
initList(newList);
copyList(newList, list);
displayList(newList);
break;
}
case 10: {
sortList(list);
cout << "排序成功!" << endl;
break;
}
case 0: {
return 0;
}
default: {
cout << "请输入正确的操作!" << endl;
break;
}
}
}
return 0;
}
```
以上就是基于线性链表实现学生通信录管理系统的示例代码,其中包含了输入、输出、插入、删除、查找、追加、读入、显示、拷贝、排序等功能。
阅读全文
相关推荐
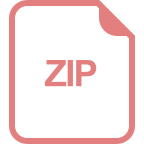

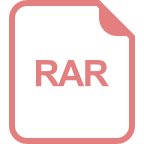
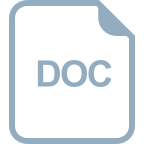
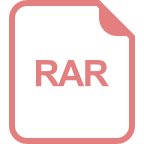
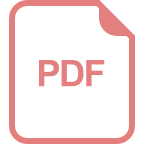
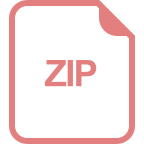
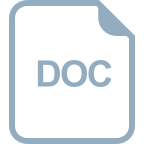
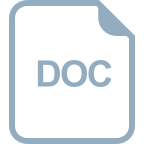
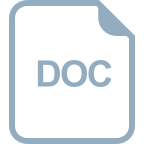
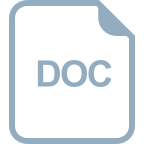
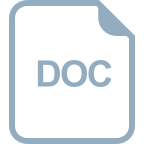
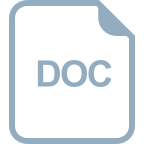
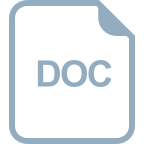
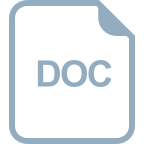
