基于Retinex理论的MSR算法代码
时间: 2024-01-21 15:20:02 浏览: 26
Retinex理论是指色彩还原理论,是一种常用于图像增强的方法。其中,MSR(Multi-scale Retinex with Color Restoration)算法是一种基于Retinex理论的图像增强算法。下面给出基于Retinex理论的MSR算法的Python实现代码。
```python
import cv2
import numpy as np
def MSR(image, sigma_list):
"""
Multi-scale Retinex with Color Restoration (MSR) algorithm
:param image: the input image
:param sigma_list: a list of sigma values for Gaussian blurring
:return: the enhanced image
"""
# Convert the input image to the YCrCb color space
ycrcb = cv2.cvtColor(image, cv2.COLOR_BGR2YCrCb)
# Split the YCrCb channels
y, cr, cb = cv2.split(ycrcb)
# Create an empty output image
output = np.zeros(image.shape, dtype=np.float32)
# Apply multi-scale Retinex algorithm to the Y channel
for sigma in sigma_list:
# Blur the image using a Gaussian filter
blur = cv2.GaussianBlur(y, (0, 0), sigma)
# Calculate the log of the blurred image
log = np.log(image + 1) - np.log(blur + 1)
# Add the log values to the output image
output += log
# Scale the output image
output = output / len(sigma_list)
# Apply color restoration to the output image
output = color_restoration(output, cr, cb)
# Convert the output image to the BGR color space
output = cv2.cvtColor(output, cv2.COLOR_YCrCb2BGR)
# Clip the pixel values to the range [0, 255]
output = np.clip(output, 0, 255)
# Convert the pixel values to the uint8 data type
output = np.uint8(output)
return output
def color_restoration(image, cr, cb):
"""
Color restoration function
:param image: the input image
:param cr: the Cr channel of the input image
:param cb: the Cb channel of the input image
:return: the output image with restored color
"""
# Calculate the mean of the input image
mean = np.mean(image)
# Create an empty output image
output = np.zeros(image.shape, dtype=np.float32)
# Apply color restoration algorithm to the image
for i in range(image.shape[0]):
for j in range(image.shape[1]):
# Calculate the chrominance values
chroma = np.sqrt((cr[i, j] ** 2) + (cb[i, j] ** 2))
# Calculate the color restoration factor
factor = np.log10(1 + mean / chroma)
# Apply the color restoration factor to the image
output[i, j] = image[i, j] * factor
return output
```
在上面的代码中,MSR函数是实现了多尺度Retinex算法的函数。该函数将输入图像转换到YCrCb颜色空间,并将其分解为Y,Cr和Cb通道。然后,它使用一个高斯滤波器对Y通道进行多尺度处理,并将所有处理后的图像叠加起来,得到增强后的Y通道。最后,它使用color_restoration函数对Y通道进行颜色恢复,并将输出图像转换回BGR颜色空间。
color_restoration函数是一个用于颜色恢复的辅助函数。该函数通过计算Cr和Cb通道的平均值和Y通道的色度值来计算颜色恢复系数,并将其应用于Y通道。最后,该函数返回颜色恢复后的图像。
使用MSR算法增强图像的示例代码如下:
```python
# Load the input image
image = cv2.imread('input.jpg')
# Apply the MSR algorithm to the image
sigma_list = [15, 80, 250]
output = MSR(image, sigma_list)
# Display the input and output images
cv2.imshow('Input', image)
cv2.imshow('Output', output)
cv2.waitKey(0)
```
在上面的代码中,我们加载了一个名为input.jpg的图像,并将其传递给MSR函数,使用sigma_list参数指定了高斯滤波器的尺度。最后,我们显示了输入图像和增强后的输出图像。
相关推荐
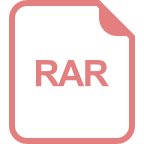














