qt opengl assimp读取ifc模型详细指导
时间: 2024-02-23 12:58:36 浏览: 218
好的,以下是更详细的指导:
1. 安装Qt、OpenGL和Assimp库
您可以从Qt官网下载并安装Qt。安装过程中,建议选择安装Qt Creator和Qt 3D Studio。
安装OpenGL库的方法因操作系统不同而有所不同。您可以在网上搜索如何安装OpenGL库的教程。
安装Assimp库的方法也因操作系统不同而有所不同。您可以在Assimp官网上找到有关如何安装Assimp库的指南。
2. 提取IFC模型的几何数据
IFC是一种基于文本的格式,为了提取IFC模型的几何数据,您可以使用IfcOpenShell库。IfcOpenShell是一个Python库,它提供了许多操作IFC模型的工具。
安装IfcOpenShell的方法请参考IfcOpenShell的官方文档。安装完成后,您可以使用以下代码来加载IFC模型:
```python
from ifcopenshell import open as ifc_open
ifc_file = ifc_open("path/to/ifc/file.ifc")
```
然后,您可以使用IfcOpenShell提供的工具来提取IFC模型的几何数据。例如,以下代码可以提取IFC模型的所有三角形面:
```python
triangles = []
for shape in ifc_file.by_type("IfcFacetedBrep"):
for face in shape.Faces:
for bound in face.Bounds:
for edge in bound.Bound:
for vertex in edge.EdgeStart.VertexGeometry:
triangles.append(vertex.Coordinates)
# 将三角形面转换为NumPy数组
import numpy as np
triangles = np.array(triangles)
```
3. 使用Assimp库将几何数据转换为OpenGL可以理解的格式
Assimp提供了许多格式转换器,包括IFC格式转换器。以下代码可以使用Assimp将IFC模型转换为OpenGL可以理解的格式:
```cpp
#include <assimp/Importer.hpp>
#include <assimp/scene.h>
#include <assimp/postprocess.h>
Assimp::Importer importer;
const aiScene* scene = importer.ReadFile("path/to/ifc/file.ifc", aiProcess_Triangulate);
// 获取模型的顶点位置、法线和纹理坐标
std::vector<float> vertices;
std::vector<float> normals;
std::vector<float> texcoords;
for (unsigned int i = 0; i < scene->mNumMeshes; i++) {
aiMesh* mesh = scene->mMeshes[i];
for (unsigned int j = 0; j < mesh->mNumVertices; j++) {
aiVector3D vertex = mesh->mVertices[j];
aiVector3D normal = mesh->mNormals[j];
aiVector3D texcoord = mesh->mTextureCoords[0][j];
vertices.push_back(vertex.x);
vertices.push_back(vertex.y);
vertices.push_back(vertex.z);
normals.push_back(normal.x);
normals.push_back(normal.y);
normals.push_back(normal.z);
texcoords.push_back(texcoord.x);
texcoords.push_back(texcoord.y);
}
}
// 获取模型的三角形面
std::vector<unsigned int> indices;
for (unsigned int i = 0; i < scene->mNumMeshes; i++) {
aiMesh* mesh = scene->mMeshes[i];
for (unsigned int j = 0; j < mesh->mNumFaces; j++) {
aiFace face = mesh->mFaces[j];
indices.push_back(face.mIndices[0]);
indices.push_back(face.mIndices[1]);
indices.push_back(face.mIndices[2]);
}
}
// 将数据传递给OpenGL
glGenBuffers(1, &vertexBuffer);
glBindBuffer(GL_ARRAY_BUFFER, vertexBuffer);
glBufferData(GL_ARRAY_BUFFER, vertices.size() * sizeof(float), &vertices[0], GL_STATIC_DRAW);
glGenBuffers(1, &normalBuffer);
glBindBuffer(GL_ARRAY_BUFFER, normalBuffer);
glBufferData(GL_ARRAY_BUFFER, normals.size() * sizeof(float), &normals[0], GL_STATIC_DRAW);
glGenBuffers(1, &texcoordBuffer);
glBindBuffer(GL_ARRAY_BUFFER, texcoordBuffer);
glBufferData(GL_ARRAY_BUFFER, texcoords.size() * sizeof(float), &texcoords[0], GL_STATIC_DRAW);
glGenBuffers(1, &indexBuffer);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, indexBuffer);
glBufferData(GL_ELEMENT_ARRAY_BUFFER, indices.size() * sizeof(unsigned int), &indices[0], GL_STATIC_DRAW);
```
请注意,上述代码仅提供了一个基本的框架,您可能需要根据您的需求进行修改。
4. 使用OpenGL渲染几何数据
以下是一个简单的OpenGL渲染代码示例:
```cpp
void GLWidget::initializeGL()
{
// 设置背景颜色
glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
// 编译和链接着色器程序
shaderProgram.addShaderFromSourceFile(QOpenGLShader::Vertex, "path/to/vertex/shader.glsl");
shaderProgram.addShaderFromSourceFile(QOpenGLShader::Fragment, "path/to/fragment/shader.glsl");
shaderProgram.link();
// 获取着色器程序中的变量位置
vertexLocation = shaderProgram.attributeLocation("vertex");
normalLocation = shaderProgram.attributeLocation("normal");
texcoordLocation = shaderProgram.attributeLocation("texcoord");
modelMatrixLocation = shaderProgram.uniformLocation("modelMatrix");
viewMatrixLocation = shaderProgram.uniformLocation("viewMatrix");
projectionMatrixLocation = shaderProgram.uniformLocation("projectionMatrix");
}
void GLWidget::resizeGL(int width, int height)
{
// 设置视口大小
glViewport(0, 0, width, height);
// 设置投影矩阵
projectionMatrix.setToIdentity();
projectionMatrix.perspective(45.0f, (float)width / height, 0.1f, 100.0f);
}
void GLWidget::paintGL()
{
// 清空缓冲区
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 启用深度测试
glEnable(GL_DEPTH_TEST);
// 使用着色器程序
shaderProgram.bind();
// 设置模型矩阵、视图矩阵和投影矩阵
shaderProgram.setUniformValue(modelMatrixLocation, modelMatrix);
shaderProgram.setUniformValue(viewMatrixLocation, viewMatrix);
shaderProgram.setUniformValue(projectionMatrixLocation, projectionMatrix);
// 启用顶点属性数组
glBindBuffer(GL_ARRAY_BUFFER, vertexBuffer);
glVertexAttribPointer(vertexLocation, 3, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(vertexLocation);
// 启用法线属性数组
glBindBuffer(GL_ARRAY_BUFFER, normalBuffer);
glVertexAttribPointer(normalLocation, 3, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(normalLocation);
// 启用纹理坐标属性数组
glBindBuffer(GL_ARRAY_BUFFER, texcoordBuffer);
glVertexAttribPointer(texcoordLocation, 2, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(texcoordLocation);
// 绘制几何数据
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, indexBuffer);
glDrawElements(GL_TRIANGLES, numIndices, GL_UNSIGNED_INT, 0);
// 禁用顶点属性数组
glDisableVertexAttribArray(vertexLocation);
glDisableVertexAttribArray(normalLocation);
glDisableVertexAttribArray(texcoordLocation);
// 解除绑定
shaderProgram.release();
glBindBuffer(GL_ARRAY_BUFFER, 0);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, 0);
}
```
这是一个简单的OpenGL渲染代码示例,您可能需要根据您的需求进行修改。
希望这些指导对您有所帮助!
阅读全文
相关推荐











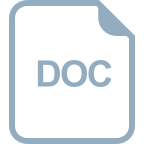




