树莓派连接百度云人脸识别怎么设计
时间: 2024-06-09 21:09:41 浏览: 11
要将树莓派与百度云人脸识别进行连接,需要以下步骤:
1. 注册百度云智能人脸识别服务并获取 API Key 和 Secret Key。
2. 在树莓派上安装 Python SDK 和 Requests 库。
3. 使用 Python 编写代码,将树莓派摄像头采集的视频流或图片上传到百度云并调用人脸识别 API 进行人脸检测和识别。检测到的人脸信息可以通过程序返回到树莓派。
4. 根据需要,可以在程序中加入其他功能,例如将识别结果通过邮件或短信发送给指定用户等。
以下是一个简单的 Python 代码示例,可以帮助你开始设计树莓派连接百度云人脸识别:
```python
import requests
import base64
# 百度云 API Key 和 Secret Key
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
# 上传图片并进行人脸检测和识别
def detect_face(image_path):
# 读取图片文件
with open(image_path, 'rb') as f:
img = base64.b64encode(f.read())
# 构造请求参数
params = {'image': img, 'face_field': 'age,gender,beauty', 'max_face_num': 5}
headers = {'Content-Type': 'application/json'}
url = 'https://aip.baidubce.com/rest/2.0/face/v3/detect?access_token=' + get_access_token()
# 发送请求
response = requests.post(url, headers=headers, json=params)
result = response.json()
# 处理返回结果
if result['error_code'] == 0:
faces = result['result']['face_list']
for face in faces:
print('性别:%s,年龄:%d,颜值:%d' % (face['gender']['type'], face['age'], face['beauty']))
else:
print('人脸检测失败:%s' % result['error_msg'])
# 获取百度云 API 的 access_token
def get_access_token():
url = 'https://aip.baidubce.com/oauth/2.0/token'
params = {'grant_type': 'client_credentials', 'client_id': API_KEY, 'client_secret': SECRET_KEY}
response = requests.post(url, params=params)
result = response.json()
return result['access_token']
# 测试代码
if __name__ == '__main__':
detect_face('test.jpg')
```
在上面的代码中,`detect_face` 函数用于上传图片并进行人脸检测和识别,`get_access_token` 函数用于获取百度云 API 的 access_token。你可以根据需要修改代码并加入其他功能。
相关推荐
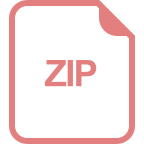
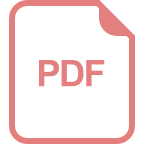
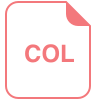
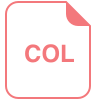
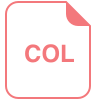

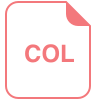









