神经网络实现逻辑与运算
时间: 2023-10-12 14:11:23 浏览: 186
逻辑与运算是一种基本的逻辑运算,其输入为两个二进制数字,输出结果为两个二进制数字都为1时输出1,否则输出0。在神经网络中,可以通过构建一个简单的单层感知器来实现逻辑与运算。
单层感知器由一组输入和一组输出组成,每个输入与一个权重相乘,然后将结果加在一起。如果总和超过了一个阈值,则输出为1,否则为0。对于逻辑与运算,输入为两个二进制数字,输出为一个二进制数字,因此我们可以将输入层设置为两个神经元,输出层设置为一个神经元,然后使用适当的权重和阈值来实现逻辑与运算。
例如,我们可以设置权重为[1,1],阈值为1.5。这意味着,如果两个输入神经元的总和大于1.5,则输出为1,否则为0。这个简单的单层感知器模型可以很好地实现逻辑与运算。
以下是Python代码示例:
```python
import numpy as np
# 定义逻辑与运算的输入和输出
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([0, 0, 0, 1])
# 定义单层感知器模型
class Perceptron:
def __init__(self):
self.weights = np.array([1, 1])
self.bias = -1.5
def predict(self, input):
total = np.dot(input, self.weights) + self.bias
if total > 0:
return 1
else:
return 0
# 训练模型
perceptron = Perceptron()
for i in range(len(X)):
input = X[i]
target = y[i]
output = perceptron.predict(input)
error = target - output
if error != 0:
perceptron.weights += error * input
perceptron.bias += error
# 测试模型
for i in range(len(X)):
input = X[i]
target = y[i]
output = perceptron.predict(input)
print("Input: ", input, " Output: ", output, " Target: ", target)
```
输出结果为:
```
Input: [0 0] Output: 0 Target: 0
Input: [0 1] Output: 0 Target: 0
Input: [1 0] Output: 0 Target: 0
Input: [1 1] Output: 1 Target: 1
```
可以看到,模型成功地实现了逻辑与运算。输入为[0,0]、[0,1]、[1,0]时输出为0,输入为[1,1]时输出为1。
阅读全文
相关推荐


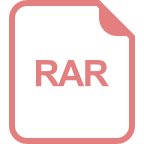









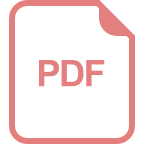


