用python写一个学生管理系统
时间: 2023-11-30 13:00:45 浏览: 36
好的,下面是一个简单的学生管理系统的python代码示例:
```python
class Student:
def __init__(self, name, id, grade):
self.name = name
self.id = id
self.grade = grade
class StudentManagementSystem:
def __init__(self):
self.students = []
def add_student(self, student):
self.students.append(student)
def remove_student(self, student_id):
for student in self.students:
if student.id == student_id:
self.students.remove(student)
def get_student(self, student_id):
for student in self.students:
if student.id == student_id:
return student
return None
def list_students(self):
for student in self.students:
print("Name: ", student.name, ", ID: ", student.id, ", Grade: ", student.grade)
# Test the student management system
system = StudentManagementSystem()
# Add some students
system.add_student(Student("Alice", "1001", "A"))
system.add_student(Student("Bob", "1002", "B"))
system.add_student(Student("Charlie", "1003", "C"))
# List all students
print("All students:")
system.list_students()
# Remove a student
system.remove_student("1002")
# List all students again
print("All students after removing Bob:")
system.list_students()
# Get a student by ID
student = system.get_student("1003")
if student:
print("Found student:", student.name)
else:
print("Student not found")
```
这个学生管理系统包含了一个 `Student` 类来表示学生,以及一个 `StudentManagementSystem` 类来管理学生。你可以在 `main()` 函数中调用这些方法来添加、删除、列出和查找学生。
相关推荐
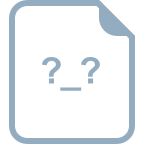
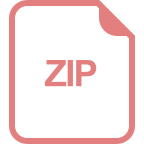
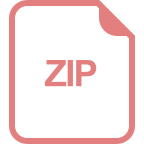




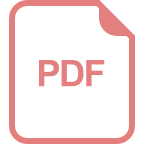
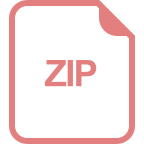
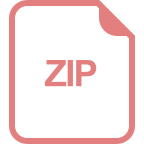
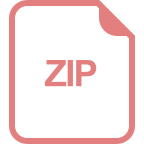
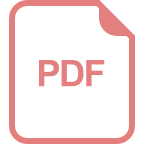
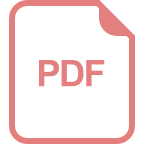
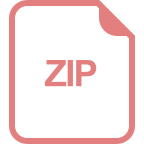
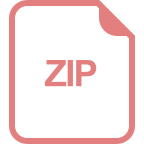