C语言实现一个静态顺序表
时间: 2024-09-15 19:10:51 浏览: 31
在C语言中,我们可以使用数组来实现一个简单的静态顺序表,也称为固定大小的顺序表,因为其元素的数量在创建时就已确定。以下是基本步骤:
```c
#include <stdio.h>
// 定义静态顺序表结构体
typedef struct StaticList {
int *data; // 存储数据的指针
int size; // 表的大小
int capacity; // 表的最大容量
int count; // 当前元素数量
} StaticList;
// 初始化静态列表
void initStaticList(StaticList *lst, int size) {
lst->data = (int*)malloc(size * sizeof(int)); // 分配内存
lst->size = size;
lst->capacity = size;
lst->count = 0;
}
// 在列表末尾添加元素
void append(StaticList *lst, int value) {
if(lst->count == lst->capacity) { // 列表满
lst->capacity *= 2; // 扩容
lst->data = (int*)realloc(lst->data, lst->capacity * sizeof(int));
}
lst->data[lst->count++] = value; // 插入元素并更新计数
}
// 获取指定索引的元素
int get(StaticList *lst, int index) {
if(index >= 0 && index < lst->count) {
return lst->data[index];
} else {
printf("Index out of bounds.\n");
return -1; // 返回错误值
}
}
// 示例
int main() {
StaticList list;
initStaticList(&list, 5);
append(&list, 10);
append(&list, 20);
printf("Element at index 0: %d\n", get(&list, 0)); // 输出10
free(list.data); // 清理内存
return 0;
}
```
相关推荐
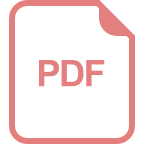















