帮我用python写一段25分钟番茄钟的代码,用上tkinter库
时间: 2024-05-02 07:20:42 浏览: 9
好的,下面是25分钟番茄钟的Python代码:
```python
import tkinter as tk
import time
def countdown(n):
while n > 0:
mins, secs = divmod(n, 60)
timeformat = '{:02d}:{:02d}'.format(mins, secs)
timer_label.config(text=timeformat)
root.update()
time.sleep(1)
n -= 1
timer_label.config(text='Time is up!')
def start_timer():
global task
task = task_entry.get()
task_entry.delete(0, tk.END)
countdown(1500) # 25 minutes = 1500 seconds
root = tk.Tk()
root.title("25 Minute Timer")
task_label = tk.Label(root, text="What task are you working on?")
task_label.pack()
task_entry = tk.Entry(root)
task_entry.pack()
start_button = tk.Button(root, text="Start Timer", command=start_timer)
start_button.pack()
timer_label = tk.Label(root, text="25:00", font=("Helvetica", 48))
timer_label.pack()
root.mainloop()
```
代码解释:
- 首先导入`tkinter`库和`time`库。
- 然后定义一个`countdown`函数,它的参数是倒计时的秒数`n`。
- 在`countdown`函数内部,使用一个`while`循环来进行倒计时,每次循环减1秒。
- 使用`divmod`函数把秒数转换为分钟和秒钟,然后按照格式化字符串的方式格式化时间。将这个时间显示在一个`Label`控件上。并调用`root.update()`函数来刷新窗口,让计时器的变化能够实时更新。
- 调用`time.sleep(1)`函数来让程序暂停1秒。
- 在循环结束后,将`timer_label`的文本改为“Time is up!”。
- 定义一个`start_timer`函数,首先使用`global`关键字声明`task`变量,然后从`task_entry`控件中获取用户输入的任务名称,并清空`task_entry`控件的内容。
- 最后调用`countdown`函数来开始计时,参数为1500,表示25分钟的秒数。
- 在主函数`root.mainloop()`中调用`pack()`方法来布局各个控件。
使用时,运行代码后在弹出的窗口中输入要进行的任务,然后点击“Start Timer”按钮即可开始计时。在25分钟结束后,弹出窗口上会显示“Time is up!”。
相关推荐
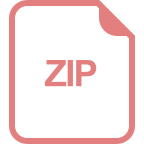
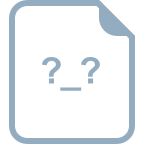
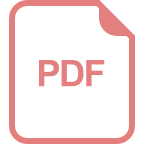
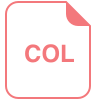
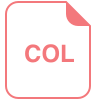
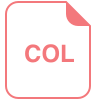
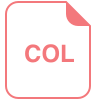
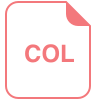









