c语言编程 统计一行字符串中单词的个数(不超过80字符)。规定所有单词由小写字母组成,单词之间有若干个空格隔开,一行的开始没有空格。
时间: 2024-02-16 19:05:00 浏览: 71
好的,以下是 C 语言代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[80];
int i, count = 1; // 初始化为1,因为一行的开始没有空格
printf("请输入一行字符串(不超过80字符):");
gets(str);
for (i = 0; i < strlen(str); i++) {
if (str[i] == ' ') {
count++; // 遇到空格,单词数加一
}
}
printf("单词数为:%d\n", count);
return 0;
}
```
这个程序中,我们使用 gets() 函数从键盘输入一行字符串,然后用一个循环遍历字符串中的每个字符。如果遇到一个空格,就说明前面的部分是一个单词,将单词数 count 加一。最后输出单词数。注意,我们在循环外面将 count 初始化为 1,因为一行的开始没有空格,是一个单词。
相关问题
用c语言编写函数fun,该函数的功能是: 统计一行字符中单词的个数,作为函数值返回。一行字符串在主函数中输入,规定所有单词由小写字母组成,单词之间由若干空格隔开,一行的开始没有空格。
以下是用c语言编写的函数fun,实现统计一行字符中单词的个数:
```c
#include <stdio.h>
int fun(char str[]) {
int count = 1;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] == ' ') {
count++;
}
}
return count;
}
int main() {
char str[100];
printf("请输入一行字符串:");
fgets(str, 100, stdin);
printf("单词个数为:%d\n", fun(str));
return 0;
}
```
首先在主函数中输入一行字符串,再将其传递给fun函数。在函数中,我们用一个计数器count来记录单词的个数,初始值为1(因为一行的开始没有空格)。接着遍历字符串,如果遇到空格,就将计数器加1。最后函数返回计数器的值,即单词的个数。
注意,这里使用fgets函数输入字符串,因为它可以避免输入太长导致缓冲区溢出的问题。同时,需要注意字符串的最大长度,这里我们假设为100。
c语言编程统计一个字符串中大写字母、小写字母、数字和其他字符的个数
好的,我很乐意为您介绍如何使用C语言编程来统计一个字符串中大写字母、小写字母、数字和其他字符的个数。以下是一个实现这个功能的C语言程序:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void countCharacters(const char *str) {
int uppercase = 0, lowercase = 0, digits = 0, others = 0;
int length = strlen(str);
for (int i = 0; i < length; i++) {
if (isupper(str[i])) {
uppercase++;
} else if (islower(str[i])) {
lowercase++;
} else if (isdigit(str[i])) {
digits++;
} else {
others++;
}
}
printf("统计结果:\n");
printf("大写字母: %d\n", uppercase);
printf("小写字母: %d\n", lowercase);
printf("数字: %d\n", digits);
printf("其他字符: %d\n", others);
}
int main() {
char str[1000];
printf("请输入一个字符串: ");
fgets(str, sizeof(str), stdin);
// 移除换行符
str[strcspn(str, "\n")] = '\0';
countCharacters(str);
return 0;
}
```
这个程序的工作原理如下:
1. 我们定义了一个`countCharacters`函数,它接受一个字符串作为参数。
2. 在这个函数中,我们使用四个变量来分别统计大写字母、小写字母、数字和其他字符的个数。
3. 我们使用`strlen`函数获取字符串的长度,然后遍历字符串中的每个字符。
4. 对于每个字符,我们使用`ctype.h`头文件中提供的函数来判断它的类型:
- `isupper`: 判断字符是否为大写字母
- `islower`: 判断字符是否为小写字母
- `isdigit`: 判断字符是否为数字
5. 根据判断结果,我们增加相应的计数器。
6. 最后,我们打印出统计结果。
7. 在`main`函数中,我们从用户那里获取输入的字符串,并调用`countCharacters`函数进行统计。
这个程序可以有效地统计字符串中各种类型字符的数量。您可以将它编译运行,并输入任意字符串来查看统计结果。
阅读全文
相关推荐
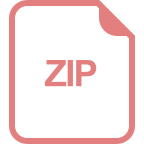














