Python如何使用OpenSSL解密AES-128数据
时间: 2024-10-28 16:13:17 浏览: 29
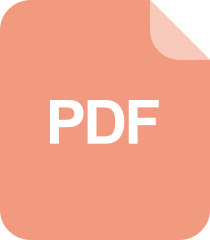
C++解密Chrome80版本数据库的方法示例代码
在Python中,你可以使用`cryptography`库,它是一个强大的加密库,支持包括OpenSSL在内的多种密码学算法。首先,你需要安装这个库,可以使用pip安装:
```bash
pip install cryptography
```
然后,你可以使用下面的步骤来解密AES-128数据:
1. 导入必要的模块:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
```
2. 加载密钥和IV(初始化向量):
```python
key = b'your_secret_key_16_bytes' # AES-128 key is 16 bytes long
iv = b'your_initialization_vector_16_bytes'
backend = default_backend()
```
3. 创建cipher对象并解密数据:
```python
cipher_text = b'encrypted_data' # 替换为你实际的加密数据
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend)
decryptor = cipher.decryptor()
decrypted_data = decryptor.update(cipher_text) + decryptor.finalize()
```
注意:如果你的数据包含前导零填充(PKCS7 padding),则需要移除这些填充。例如,可以使用`cryptography`库的`pad`和`unpad`函数。
完整示例:
```python
import base64
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
# ... (加载密钥和IV)
# Base64解码如果数据是Base64编码
cipher_text_base64 = 'base64-encoded-ciphertext'
cipher_text = base64.b64decode(cipher_text_base64)
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=default_backend())
decryptor = cipher.decryptor()
# 如果有填充,则去除填充
padded_decrypted_data = decryptor.update(cipher_text) + decryptor.finalize()
unpadded_decrypted_data = padded_decrypted_data[:-ord(padded_decrypted_data[-1])]
print("Decrypted Data:", unpadded_decrypted_data)
```
阅读全文
相关推荐
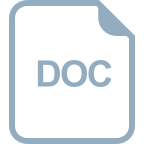
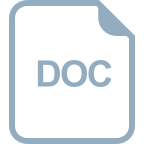
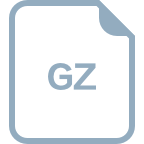
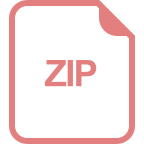
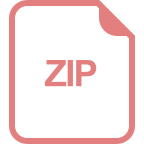
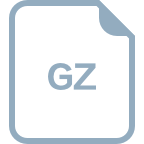
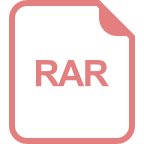
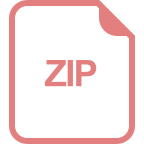
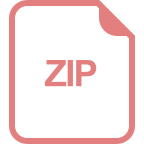
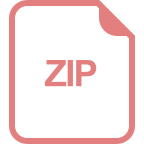
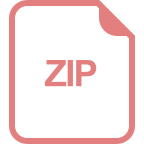
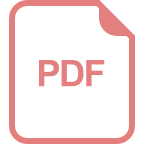
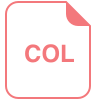
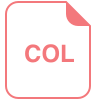
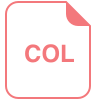
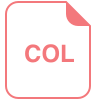
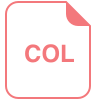
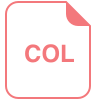