C语言实现使用原始套接字获取网口arp报文并解析
时间: 2024-10-19 15:16:02 浏览: 52
在C语言中,通过原始套接字(raw sockets)获取网络接口的ARP报文并解析通常涉及到以下几个步骤:
1. **包括头文件**:
首先需要包含一些必要的头文件,如 `<netinet/in.h>`、`<arpa/inet.h>` 和 `<sys/socket.h>`。
```c
#include <netinet/in.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <linux/if_ether.h> // 对于Ethernet ARP
```
2. **创建原始套接字**:
使用 `socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ARP))` 创建一个原始套接字,其中`AF_PACKET` 表示网络数据包,`SOCK_RAW` 表示原始协议套接字,`ETH_P_ARP` 指定我们想要接收的是ARP报文。
```c
int sock = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ARP));
if (sock < 0) {
perror("Error creating raw socket");
}
```
3. **设置接口地址**:
为了指定监听特定网卡,可以使用 `bind()` 函数将套接字绑定到相应的设备名或接口ID。
```c
struct sockaddr_ll addr;
memset(&addr, 0, sizeof(addr));
addr.sll_family = AF_PACKET; // Ethernet address family
// 如果你知道网卡名字,可以用 getifaddrs() 获取对应的索引
// 或者直接设置硬件地址,如 for Linux:
addr.sll_addr = if_nametoindex("eth0") == IFNAMSPEC ? NULL : dev->ifr_addr;
bind(sock, (struct sockaddr *)&addr, sizeof(addr));
```
4. **接收和解析ARP报文**:
使用 `recvfrom()` 接收数据包,并将其解码成结构体进行处理。例如,`struct arphdr` 可用于存储 ARP 报文信息。
```c
struct arphdr arp_hdr;
struct ether_header eth_hdr;
char packet[1500];
unsigned char *data = packet;
while (true) {
int bytes_received = recvfrom(sock, packet, sizeof(packet), 0, NULL, NULL);
if (bytes_received <= 0) {
perror("Error receiving data");
break;
}
// 解析ARP报文
memcpy(ð_hdr, packet, sizeof(struct ether_header));
struct iphdr *iph = (struct iphdr*)(packet + ETH_HLEN);
if (iph->protocol == IPPROTO_ICMP)
continue; // 不处理ICMP
struct arphdr* arp = (struct arphdr*)(packet + eth_hdr.hlen);
/* 解析和处理 ARP 包的具体内容 */
}
```
5. **处理报文**:
在 `arp` 结构体内,你可以提取源IP地址、目标IP地址、硬件地址等信息,并根据需求进一步操作。
注意:以上代码仅适用于Linux系统,其他操作系统可能会有不同的API和细节。此外,使用原始套接字可能涉及权限管理,确保有足够的权限访问网络接口。
阅读全文
相关推荐
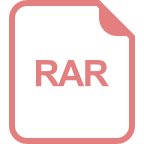
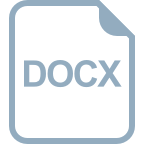
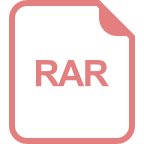
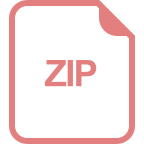
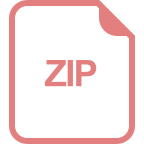
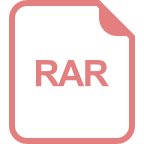
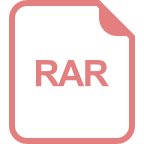
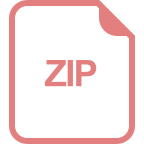
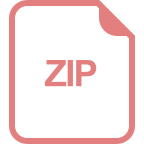
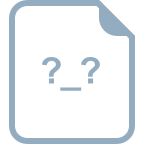
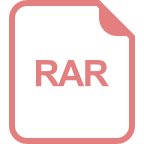
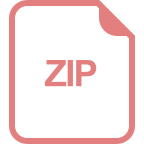
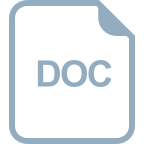