将两个递增有序链表合并为一个递增有序链表。要求 // 1.结果链表仍然使用原来两个链表的存储空间,不占用其它空间 // 2.表中不许有重复数据,用c语言完整写出来
时间: 2024-09-23 20:14:59 浏览: 50
将两个递增有序链表合并成一个新的递增有序链表,可以使用迭代的方式解决,同时保持链表原有的内存分配,并避免插入重复元素。下面是一个C语言的解决方案:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int val; // 节点值
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
// 创建新节点
ListNode* createNode(int val) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode != NULL) {
newNode->val = val;
newNode->next = NULL;
}
return newNode;
}
// 合并两个有序链表
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode* dummy = createNode(0); // 创建虚拟头节点
ListNode* tail = dummy; // 尾节点指向虚拟头
while (l1 != NULL && l2 != NULL) {
if (l1->val <= l2->val) {
tail->next = l1;
l1 = l1->next;
} else {
tail->next = l2;
l2 = l2->next;
}
tail = tail->next;
}
// 如果其中一个链表未遍历完,将其剩余部分添加到结果链表尾部
if (l1 != NULL)
tail->next = l1;
else if (l2 != NULL)
tail->next = l2;
return dummy->next; // 返回实际的头部节点
}
// 打印链表
void printList(ListNode* head) {
while (head != NULL) {
printf("%d -> ", head->val);
head = head->next;
}
printf("NULL\n");
}
int main() {
// 测试示例
ListNode* l1 = createNode(1);
l1->next = createNode(2);
l1->next->next = createNode(4);
ListNode* l2 = createNode(1);
l2->next = createNode(3);
l2->next->next = createNode(4);
ListNode* result = mergeTwoLists(l1, l2);
printList(result); // 输出:1 -> 1 -> 2 -> 3 -> 4 -> 4 -> NULL
return 0;
}
```
这个程序首先创建一个虚拟头节点,然后通过对比两个链表的当前节点值来决定添加哪一个到结果链表中。最后返回合并后的实际链表头部。
阅读全文
相关推荐
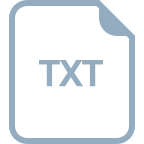
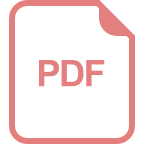
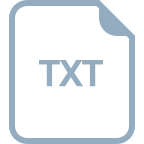
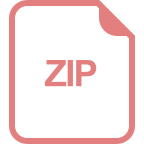
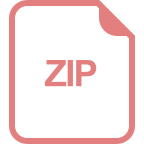
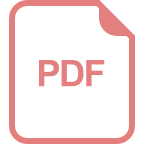
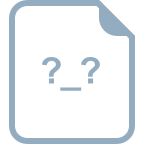
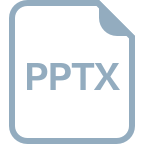
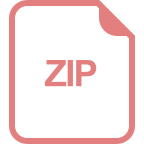
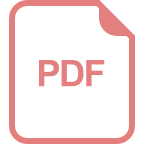