if (productData.isEmpty.All(b=>b)) { }
时间: 2024-09-18 19:08:59 浏览: 56
这个`if`条件表达式是一个常见的LINQ查询,其中`productData.isEmpty.All(b => b)`是用来检查一个名为`productData`的集合(在这里假设是`List<Product>`或者其他支持`isEmpty`和`All`操作的集合),其`isEmpty`属性表示该集合是否为空,而`All(b => b)`则检查每一个元素`b`是否满足某个布尔条件(这里假设`b`代表每个产品数据`Product`的某个布尔字段)。
如果`productData.isEmpty`为`true`并且所有`Product`实例的相应布尔字段也全部为`true`,那么`All(b => b)`会返回`true`,`if`语句内的代码块将会被执行;反之,如果有一个元素不满足布尔条件或者集合本身为空,`All`函数就会返回`false`,`if`语句内部的代码块就不会执行。
举个例子,如果`Product`有一个名为`IsActive`的字段,你可以这么检查:
```csharp
if (productData.isEmpty && productData.All(p => !p.IsActive)) {
// 执行一些代码,因为所有产品都已停用且列表为空
}
```
相关问题
switch (goodsItem.getProductType()) { case 1://爆品 GoodsExplosive explosive = null; List<GoodsExplosive> explosives = new GoodsExplosive().selectList(new LambdaUpdateWrapper<GoodsExplosive>() .eq(GoodsExplosive::getGoodsItemId, goodsItem.getId()) .orderByDesc(GoodsExplosive::getId)); if (explosives.size() > 1) { explosive = explosives.stream().sorted(Comparator.comparing(GoodsExplosive::getGroundingState, (s1, s2) -> { if (s1.equals(s2)) { return 0; } else if (s1 == 1 || s2 == 5) { return -1; } else if (s1 == 5 || s2 == 1) { return 1; } else if (s1 == 2) { return -1; } else if (s2 == 2) { return 1; } else if (s1 == 0) { return -1; } else { return 1; } })).findFirst().orElse(null); } else { explosive = explosives.size() == 1 ? explosives.get(0) : explosive; } map.put("productData", explosive); break; case 2:// GoodsExcellent excellent = null; List<GoodsExcellent> excellents = new GoodsExcellent().selectList(new LambdaUpdateWrapper<GoodsExcellent>() .eq(GoodsExcellent::getGoodsItemId, goodsItem.getId()) .orderByDesc(GoodsExcellent::getId)); if (excellents.size() > 1) { excellent = excellents.stream().sorted(Comparator.comparing(GoodsExcellent::getGroundingState, (s1, s2) -> { if (s1.equals(s2)) { return 0; } else if (s1 == 1 || s2 == 5) { return -1; } else if (s1 == 5 || s2 == 1) { return 1; } else if (s1 == 2) { return -1; } else if (s2 == 2) { return 1; } else if (s1 == 0) { return -1; } else { return 1; } })).findFirst().orElse(null); } else { excellent = excellents.size() == 1 ? excellents.get(0) : excellent; } map.put("productData", excellent); break; case 3://现货 GoodsEnjoy enjoy = null; List<GoodsEnjoy> enjoys = new GoodsEnjoy().selectList(new LambdaUpdateWrapper<GoodsEnjoy>() .eq(GoodsEnjoy::getGoodsItemId, goodsItem.getId() .orderByDesc(GoodsEnjoy::getId)); if (enjoys.size() > 1) { enjoy = enjoys.stream().sorted(Comparator.comparing(GoodsEnjoy::getGroundingState, (s1, s2) -> { if (s1.equals(s2)) { return 0; } else if (s1 == 1 || s2 == 5) { return -1; } else if (s1 == 5 || s2 == 1) { return 1; } else if (s1 == 2) { return -1; } else if (s2 == 2) { return 1; } else if (s1 == 0) { return -1; } else { return 1; } })).findFirst().orElse(null); } else { enjoy = enjoys.size() == 1 ? enjoys.get(0) : enjoy; } map.put("productData", enjoy); break; default: throw new IllegalStateException("Unexpected value: " + goodsItem.getProductType()); }怎么优化
这段代码中有重复的逻辑,可以抽象成一个方法,减少重复代码。同时,可以使用 Java 8 的 Optional 等新特性简化代码。具体来说,可以将以下部分重构:
```
GoodsExplosive explosive = null;
List<GoodsExplosive> explosives = new GoodsExplosive().selectList(new LambdaUpdateWrapper<GoodsExplosive>() .eq(GoodsExplosive::getGoodsItemId, goodsItem.getId()) .orderByDesc(GoodsExplosive::getId));
if (explosives.size() > 1) {
explosive = explosives.stream().sorted(Comparator.comparing(GoodsExplosive::getGroundingState, (s1, s2) -> {
if (s1.equals(s2)) {
return 0;
} else if (s1 == 1 || s2 == 5) {
return -1;
} else if (s1 == 5 || s2 == 1) {
return 1;
} else if (s1 == 2) {
return -1;
} else if (s2 == 2) {
return 1;
} else if (s1 == 0) {
return -1;
} else {
return 1;
}
})).findFirst().orElse(null);
} else {
explosive = explosives.size() == 1 ? explosives.get(0) : explosive;
}
```
可以抽象成一个方法:
```
private Optional<GoodsExplosive> getGoodsExplosive(GoodsItem goodsItem) {
List<GoodsExplosive> explosives = new GoodsExplosive().selectList(new LambdaUpdateWrapper<GoodsExplosive>()
.eq(GoodsExplosive::getGoodsItemId, goodsItem.getId())
.orderByDesc(GoodsExplosive::getId));
return explosives.stream().sorted(Comparator.comparing(GoodsExplosive::getGroundingState, (s1, s2) -> {
if (s1.equals(s2)) {
return 0;
} else if (s1 == 1 || s2 == 5) {
return -1;
} else if (s1 == 5 || s2 == 1) {
return 1;
} else if (s1 == 2) {
return -1;
} else if (s2 == 2) {
return 1;
} else if (s1 == 0) {
return -1;
} else {
return 1;
}
})).findFirst();
}
```
然后可以简化 switch 语句:
```
switch (goodsItem.getProductType()) {
case 1: //爆品
map.put("productData", getGoodsExplosive(goodsItem).orElse(null));
break;
case 2: //精品
map.put("productData", new GoodsExcellent().selectList(new LambdaUpdateWrapper<GoodsExcellent>()
.eq(GoodsExcellent::getGoodsItemId, goodsItem.getId())
.orderByDesc(GoodsExcellent::getId))
.stream().findFirst().orElse(null));
break;
case 3: //现货
map.put("productData", new GoodsEnjoy().selectList(new LambdaUpdateWrapper<GoodsEnjoy>()
.eq(GoodsEnjoy::getGoodsItemId, goodsItem.getId())
.orderByDesc(GoodsEnjoy::getId))
.stream().findFirst().orElse(null));
break;
default:
throw new IllegalStateException("Unexpected value: " + goodsItem.getProductType());
}
```
解释下列代码package sparkML import org.apache.spark.ml.clustering.{KMeans, KMeansModel} import org.apache.spark.ml.feature.{HashingTF, IDF, Tokenizer} import org.apache.spark.sql.{DataFrame, SparkSession} object Kmeans { case class Product(id: String, wd: String, jd: String, sjc: String, jyje: String) def main(args: Array[String]): Unit = { val spark = SparkSession .builder() .appName("TFIDF@2") .master("local[3]") .getOrCreate() import spark.implicits._ val product = spark.sparkContext.textFile("data/data.txt").map { x => val data = x.split(",") Product(data(0), data(1), data(2), data(3), data(4)) }.toDS().cache() product.createOrReplaceTempView("data") val products: DataFrame = spark.sql("select id, concat_ws(',',collect_set(jyje)) as jyje from data group by id") val productData = new Tokenizer().setInputCol("jyje").setOutputCol("productWords").transform(products) val tfData = new HashingTF().setNumFeatures(20).setInputCol("productWords").setOutputCol("productFeatures").transform(productData) val idfModel = new IDF().setInputCol("productFeatures").setOutputCol("features").fit(tfData) val idfData = idfModel.transform(tfData) val trainingData = idfData.select("id", "features") val kmeans = new KMeans() .setK(4) .setMaxIter(5) .setFeaturesCol("features") .setPredictionCol("prediction") val kmeansModel = kmeans.fit(trainingData) val kmeansData = kmeansModel.transform(trainingData) kmeansData.show() } }
这段代码是一个使用Spark ML库实现KMeans聚类算法的示例。代码中定义了一个名为Product的case class,表示一个产品的属性集合。代码中首先使用SparkContext的textFile方法读取数据文件,然后使用map方法将每行数据转换为Product对象,并转换成DataFrame格式。接着,使用Spark SQL对DataFrame进行分组和聚合操作,得到每个产品的交易金额数据。然后,使用Tokenizer和HashingTF对文本进行分词和特征提取,使用IDF对特征进行加权,得到最终的训练数据。最后,使用KMeans算法对训练数据进行聚类,并输出聚类结果。
阅读全文
相关推荐
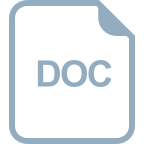
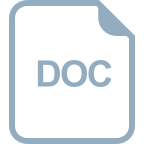
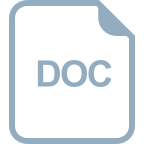
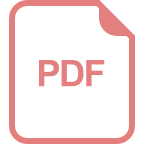
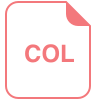
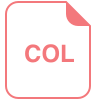








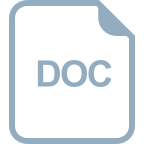
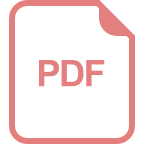