如何在C#中将图片转换为Bitmap类型?
时间: 2024-10-20 07:17:47 浏览: 54
在C#中,将图片转换为Bitmap类型可以通过多种方式实现。以下是一些常见的方法:
1. **从文件加载**:使用`Image.FromFile`方法从文件中加载图像,并将其转换为`Bitmap`。
```csharp
using System.Drawing;
Bitmap bitmap = new Bitmap("path_to_image_file");
```
2. **从字节数组加载**:如果你有一个包含图像数据的字节数组,可以使用`Image.FromStream`方法将其转换为`Bitmap`。
```csharp
byte[] imageBytes = // your byte array containing the image data
using (MemoryStream ms = new MemoryStream(imageBytes))
{
Bitmap bitmap = new Bitmap(Image.FromStream(ms));
}
```
3. **从网络流加载**:从网络URL获取图像数据并转换为`Bitmap`。
```csharp
using System.Net;
using System.IO;
using System.Drawing;
WebClient webClient = new WebClient();
byte[] imageBytes = webClient.DownloadData("image_url");
using (MemoryStream ms = new MemoryStream(imageBytes))
{
Bitmap bitmap = new Bitmap(Image.FromStream(ms));
}
```
4. **从资源加载**:如果你的项目中包含图像资源,可以直接从资源中加载图像。
```csharp
Properties.Resources.YourImageResource.ToBitmap();
```
5. **从其他图像类型转换**:如果已有其他类型的图像对象(如`Image`),可以强制转换为`Bitmap`。
```csharp
Image image = Image.FromFile("path_to_image_file");
Bitmap bitmap = new Bitmap(image);
```
阅读全文
相关推荐
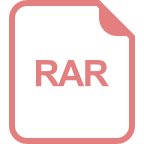
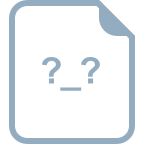
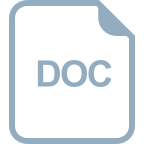



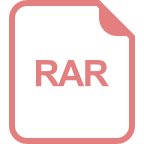
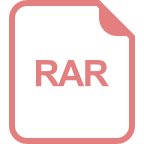
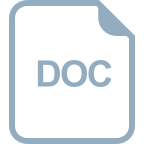
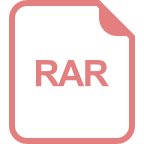
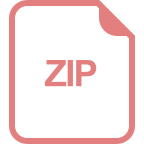
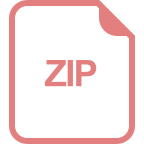
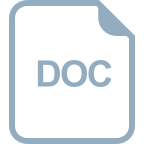





