用C语言实现银行家算法避免死锁
时间: 2023-11-18 19:04:46 浏览: 36
银行家算法是一种避免死锁的算法,它通过动态地分配资源来避免死锁的发生。下面是一个用C语言实现银行家算法的示例:
```c
#include <stdio.h>
#include <stdbool.h>
#define P 5 // 进程数
#define R 3 // 资源数
int available[R] = {3, 3, 2}; // 可用资源数
int max[P][R] = {{7, 5, 3}, {3, 2, 2}, {9, 0, 2}, {2, 2, 2}, {4, 3, 3}}; // 最大需求矩阵
int allocation[P][R] = {{0, 1, 0}, {2, 0, 0}, {3, 0, 2}, {2, 1, 1}, {0, 0, 2}}; // 已分配矩阵
int need[P][R]; // 需求矩阵
bool finish[P] = {false}; // 进程是否完成
void calculate_need() {
for (int i = 0; i < P; i++) {
for (int j = 0; j < R; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
}
bool is_safe() {
int work[R];
for (int i = 0; i < R; i++) {
work[i] = available[i];
}
bool finish_all = false;
while (!finish_all) {
bool can_allocate = false;
for (int i = 0; i < P; i++) {
if (!finish[i]) {
bool can_finish = true;
for (int j = 0; j < R; j++) {
if (need[i][j] > work[j]) {
can_finish = false;
break;
}
}
if (can_finish) {
can_allocate = true;
finish[i] = true;
for (int j = 0; j < R; j++) {
work[j] += allocation[i][j];
}
}
}
}
if (!can_allocate) {
for (int i = 0; i < P; i++) {
if (!finish[i]) {
return false;
}
}
finish_all = true;
}
}
return true;
}
void request_resources(int process_id, int request[]) {
for (int i = 0; i < R; i++) {
if (request[i] > need[process_id][i] || request[i] > available[i]) {
printf("Error: Requested resources exceed need or available.\n");
return;
}
}
for (int i = 0; i < R; i++) {
available[i] -= request[i];
allocation[process_id][i] += request[i];
need[process_id][i] -= request[i];
}
if (is_safe()) {
printf("Request approved. System is safe.\n");
} else {
printf("Request denied. System will be unsafe.\n");
for (int i = 0; i < R; i++) {
available[i] += request[i];
allocation[process_id][i] -= request[i];
need[process_id][i] += request[i];
}
}
}
int main() {
calculate_need();
int request[P][R] = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}, {0, 0, 0}, {0, 0, 0}}; // 请求资源矩阵
int process_id;
printf("Enter process ID (0-4): ");
scanf("%d", &process_id);
printf("Enter resource request (e.g. 1 0 2): ");
for (int i = 0; i < R; i++) {
scanf("%d", &request[process_id][i]);
}
request_resources(process_id, request[process_id]);
return 0;
}
```
以上代码实现了银行家算法的主要功能,包括计算需求矩阵、判断系统是否安全、请求资源等。用户可以根据自己的需求修改进程数、资源数、可用资源数、最大需求矩阵、已分配矩阵等参数,以及根据需要添加其他功能。
相关推荐
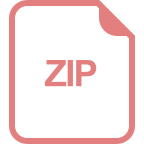
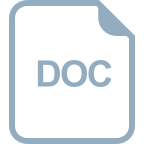














