c#WPF上机考试试题
时间: 2024-12-28 22:24:16 浏览: 10
### C# WPF 实践考试题目
#### 题目一:创建一个简单的WPF应用程序
设计并实现一个名为`SimpleApp`的简单WPF应用程序,该程序应具有以下功能:
- 应用程序窗口标题为“简易计算器”
- 窗口内有一个TextBox用于输入数字[^1]
- 提供两个按钮:“加法”和“减法”,点击相应按钮可以执行相应的算术运算并将结果显示在同一TextBox中[^2]
```csharp
using System;
using System.Windows;
namespace SimpleApp {
public partial class MainWindow : Window {
private double currentValue = 0;
public MainWindow() {
InitializeComponent();
}
private void AddButton_Click(object sender, RoutedEventArgs e) {
if (double.TryParse(textBox.Text, out var number)) {
currentValue += number;
textBox.Clear();
ResultTextBlock.Text = $"Result: {currentValue}";
}
}
private void SubtractButton_Click(object sender, RoutedEventArgs e) {
if (double.TryParse(textBox.Text, out var number)) {
currentValue -= number;
textBox.Clear();
ResultTextBlock.Text = $"Result: {currentValue}";
}
}
}
}
```
#### 题目二:数据绑定与命令模式的应用
构建一个能够展示员工信息列表的小型应用。此项目需满足如下条件:
- 使用MVVM架构来分离视图逻辑和业务逻辑[^3]
- 利用ObservableCollection存储多个Employee对象实例,并通过XAML中的ItemsControl控件显示这些条目的集合[^4]
- 定义ICommand接口的具体实现类RelayCommand以便于处理UI事件触发的操作[^5]
```xml
<!-- EmployeeView.xaml -->
<Window x:Class="EmployeesApp.EmployeeView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation">
<Grid>
<!-- 绑定到ViewModel的数据源 -->
<ListBox ItemsSource="{Binding Employees}">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding Name}" />
<TextBlock Text=", " />
<TextBlock Text="{Binding Position}" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<Button Content="Add New" Command="{Binding AddNewEmployeeCommand}"/>
</Grid>
</Window>
```
阅读全文
相关推荐


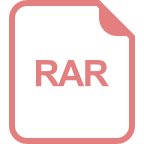

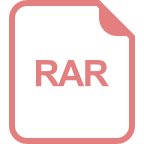
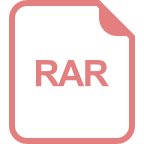
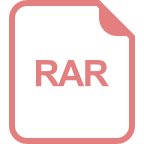
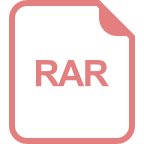
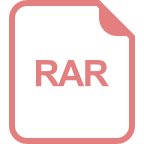

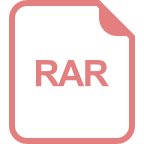
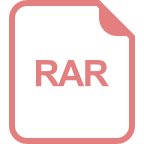
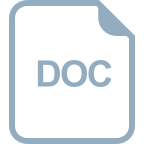
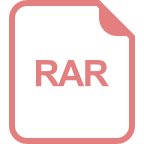

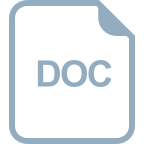
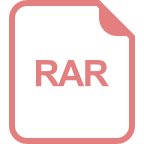

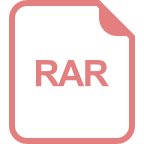