python des加密
时间: 2023-10-29 07:57:35 浏览: 188
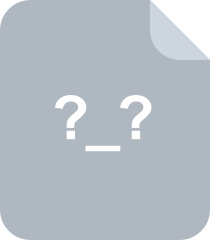
Python Des加密
Python中可以使用DES加密算法进行加密。你可以通过创建一个DES类的实例来实现加密和解密操作。首先,你需要初始化DES的各种置换表和默认密钥。然后,你可以使用str2bin函数将明文转换为二进制字符串。接下来,你可以使用encode函数对明文进行加密。最后,你可以使用bin2str函数将密文转换回字符串。
下面是一个示例代码,演示了如何使用Python实现DES加密:
```python
import binascii
from Cryptodome.Cipher import DES
class DES:
def __init__(self):
self.key = b"12345678" # 设置默认密钥
def str2bin(self, string):
binary = bin(int(binascii.hexlify(string.encode()), 16))[2:] # 将明文转为二进制字符串
return binary
def bin2str(self, binary):
string = binascii.unhexlify(hex(int(binary, 2))[2:]).decode() # 二进制字符串转成字符串
return string
def encode(self, plaintext):
cipher = DES.new(self.key, DES.MODE_ECB) # 创建DES对象
ciphertext = cipher.encrypt(plaintext.encode()) # 加密
return binascii.hexlify(ciphertext).decode()
def decode(self, ciphertext):
cipher = DES.new(self.key, DES.MODE_ECB) # 创建DES对象
plaintext = cipher.decrypt(binascii.unhexlify(ciphertext)).decode() # 解密
return plaintext
def main(self):
plaintext = "Hello, DES!" # 明文
binary = self.str2bin(plaintext) # 明文转为二进制字符串
ciphertext = self.encode(plaintext) # 加密
decrypted_text = self.decode(ciphertext) # 解密
print("明文:", plaintext)
print("明文的二进制表示:", binary)
print("密文:", ciphertext)
print("解密后的明文:", decrypted_text)
des = DES()
des.main()
```
上述代码中,我们使用了Cryptodome库中的DES模块。首先,我们创建了一个DES类,其中包含了str2bin、bin2str、encode、decode和main等方法。str2bin方法将明文转换为二进制字符串,bin2str方法将二进制字符串转换为字符串。encode方法使用默认密钥对明文进行加密,decode方法使用默认密钥对密文进行解密。main方法是一个测试函数,用于演示加密和解密的过程。
希望对你有所帮助!
阅读全文
相关推荐



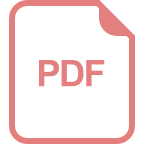
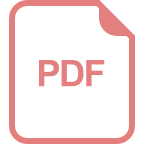
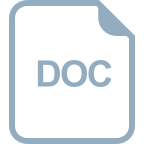
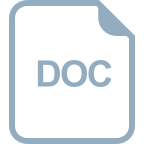
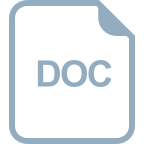
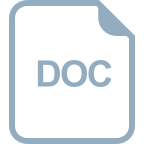



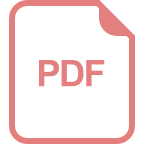