用VHDL语言编写一个五选一开关程序,要求编写三个结构体,分别用条件信号赋值语句,IF语句,CASE语句的方式实现。其中A,B,C,D,E端口均为8bit输入信号,S为3bit选择信号,Y为8bit输出信号
时间: 2024-05-12 09:18:55 浏览: 61
1. 条件信号赋值语句实现:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
entity mux_5to1 is
port (
A, B, C, D, E: in std_logic_vector(7 downto 0);
S: in std_logic_vector(2 downto 0);
Y: out std_logic_vector(7 downto 0)
);
end entity;
architecture cond_assignment of mux_5to1 is
begin
Y <= A when (S = "000") else
B when (S = "001") else
C when (S = "010") else
D when (S = "011") else
E;
end architecture;
```
2. IF语句实现:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
entity mux_5to1 is
port (
A, B, C, D, E: in std_logic_vector(7 downto 0);
S: in std_logic_vector(2 downto 0);
Y: out std_logic_vector(7 downto 0)
);
end entity;
architecture if_statement of mux_5to1 is
begin
process (A, B, C, D, E, S)
begin
if S = "000" then
Y <= A;
elsif S = "001" then
Y <= B;
elsif S = "010" then
Y <= C;
elsif S = "011" then
Y <= D;
else
Y <= E;
end if;
end process;
end architecture;
```
3. CASE语句实现:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
entity mux_5to1 is
port (
A, B, C, D, E: in std_logic_vector(7 downto 0);
S: in std_logic_vector(2 downto 0);
Y: out std_logic_vector(7 downto 0)
);
end entity;
architecture case_statement of mux_5to1 is
begin
process (S)
begin
case S is
when "000" =>
Y <= A;
when "001" =>
Y <= B;
when "010" =>
Y <= C;
when "011" =>
Y <= D;
when others =>
Y <= E;
end case;
end process;
end architecture;
```
阅读全文
相关推荐
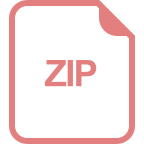
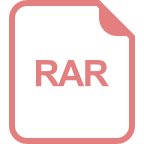
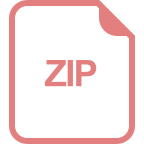















