用Java写一个日历加钟表要带图形界面
时间: 2024-06-02 17:09:10 浏览: 14
要实现一个日历加钟表的图形界面,可以使用Java Swing或JavaFX框架来开发。以下是一个简单的实现示例:
1. 创建一个Java项目,并导入Swing或JavaFX库。
2. 创建一个主窗口,可使用JFrame或Stage类。
3. 在窗口中添加一个日历和一个钟表组件。可以使用JCalendar库来创建日历组件,使用时钟类来创建钟表组件。
4. 将日历和钟表组件放置在窗口中,可以使用布局管理器来控制它们的位置和大小。
5. 为日历和钟表添加事件监听器,以便在用户进行操作时更新它们的显示。
6. 运行程序并测试。
以下是一个Java Swing实现示例代码:
```java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingConstants;
import javax.swing.Timer;
import com.toedter.calendar.JCalendar;
public class CalendarClock extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JPanel calendarPanel, clockPanel;
private JCalendar calendar;
private JLabel timeLabel, dateLabel;
private Timer timer;
public CalendarClock() {
// 设置窗口属性
setTitle("CalendarClock");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setLocationRelativeTo(null);
// 创建日历组件
calendarPanel = new JPanel(new BorderLayout());
calendar = new JCalendar();
calendarPanel.add(calendar, BorderLayout.CENTER);
// 创建钟表组件
clockPanel = new JPanel(new GridLayout(2, 1));
timeLabel = new JLabel("", SwingConstants.CENTER);
timeLabel.setFont(new Font("Arial", Font.BOLD, 24));
timeLabel.setForeground(Color.BLUE);
dateLabel = new JLabel("", SwingConstants.CENTER);
dateLabel.setFont(new Font("Arial", Font.BOLD, 18));
dateLabel.setForeground(Color.RED);
clockPanel.add(timeLabel);
clockPanel.add(dateLabel);
// 添加事件监听器
calendar.getDayChooser().addPropertyChangeListener("day", this);
timer = new Timer(1000, this);
timer.start();
// 将日历和钟表组件放置在窗口中
add(calendarPanel, BorderLayout.WEST);
add(clockPanel, BorderLayout.CENTER);
// 显示窗口
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
// 更新钟表组件显示
Date now = new Date();
SimpleDateFormat timeFormat = new SimpleDateFormat("HH:mm:ss");
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
timeLabel.setText(timeFormat.format(now));
dateLabel.setText(dateFormat.format(now));
}
public static void main(String[] args) {
new CalendarClock();
}
}
```
运行程序后,将显示如下图形界面:

相关推荐
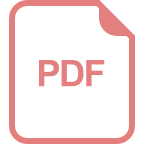
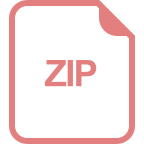













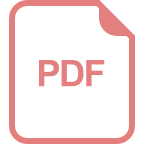