不使用opencv,用c语言提取bmp图像RGB值
时间: 2023-05-19 15:03:49 浏览: 252
可以使用C语言的标准库函数来提取BMP图像的RGB值。首先需要读取BMP文件头信息,然后根据文件头信息中的宽度和高度信息,逐行读取像素数据,再根据像素数据中的颜色分量信息提取RGB值。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#pragma pack(push, 1)
typedef struct {
uint16_t type;
uint32_t size;
uint16_t reserved1;
uint16_t reserved2;
uint32_t offset;
} bmp_file_header_t;
typedef struct {
uint32_t size;
int32_t width;
int32_t height;
uint16_t planes;
uint16_t bit_count;
uint32_t compression;
uint32_t size_image;
int32_t x_pels_per_meter;
int32_t y_pels_per_meter;
uint32_t clr_used;
uint32_t clr_important;
} bmp_info_header_t;
#pragma pack(pop)
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s <bmp_file>\n", argv[0]);
return 1;
}
FILE *fp = fopen(argv[1], "rb");
if (!fp) {
printf("Failed to open file: %s\n", argv[1]);
return 1;
}
bmp_file_header_t file_header;
bmp_info_header_t info_header;
fread(&file_header, sizeof(file_header), 1, fp);
fread(&info_header, sizeof(info_header), 1, fp);
if (file_header.type != 0x4D42) {
printf("Invalid BMP file format\n");
return 1;
}
if (info_header.bit_count != 24) {
printf("Unsupported color depth: %d\n", info_header.bit_count);
return 1;
}
int width = info_header.width;
int height = info_header.height;
int row_size = (width * 3 + 3) & ~3; // BMP行字节数为4的倍数
uint8_t *data = malloc(row_size * height);
if (!data) {
printf("Failed to allocate memory\n");
return 1;
}
fseek(fp, file_header.offset, SEEK_SET);
fread(data, row_size, height, fp);
fclose(fp);
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
uint8_t *pixel = data + y * row_size + x * 3;
uint8_t r = pixel[2];
uint8_t g = pixel[1];
uint8_t b = pixel[0];
printf("(%d,%d): R=%d, G=%d, B=%d\n", x, y, r, g, b);
}
}
free(data);
return 0;
}
```
这个程序可以读取一个BMP文件,并输出每个像素的RGB值。注意,由于BMP文件的行字节数为4的倍数,因此需要使用位运算来计算每行的字节数。
阅读全文
相关推荐
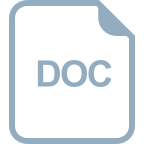
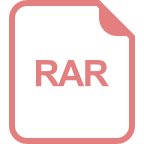
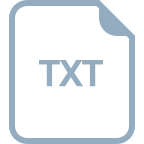
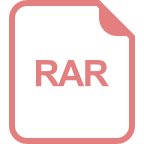
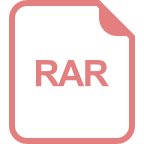
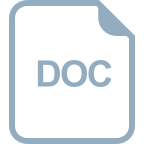
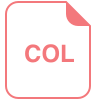

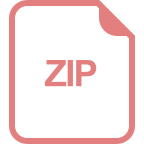
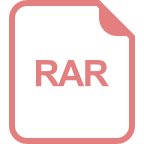
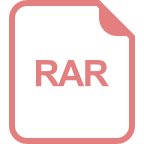
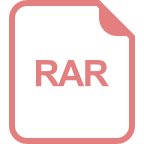
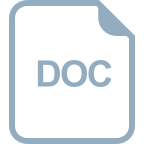
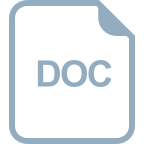
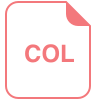
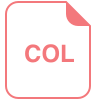