1.编写“求任意两个正整数的最大公约数“算法,使用make维护。要求如下 (1)编写makefile文件,将main.c编 译成可执行目标文件main,并运行main; (2)包含gcd.c和output.c完成计算和输出功能
时间: 2023-06-20 22:10:20 浏览: 61
下面是一个可能的实现方法:
1. 首先编写gcd.c文件,实现求最大公约数函数:
```c
int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
```
2. 接着编写output.c文件,实现输出结果的函数:
```c
#include <stdio.h>
void print_gcd(int a, int b, int result) {
printf("The gcd of %d and %d is %d\n", a, b, result);
}
```
3. 编写makefile文件,将上述两个文件和main.c文件编译成可执行目标文件:
```makefile
CC=gcc
CFLAGS=-I.
DEPS = gcd.h
OBJ = gcd.o output.o main.o
%.o: %.c $(DEPS)
$(CC) -c -o $@ $< $(CFLAGS)
main: $(OBJ)
$(CC) -o $@ $^ $(CFLAGS)
```
4. 最后编写main.c文件,调用上述函数并输出结果:
```c
#include "gcd.h"
#include "output.h"
int main() {
int a = 12, b = 18;
int result = gcd(a, b);
print_gcd(a, b, result);
return 0;
}
```
5. 在终端中执行make命令即可编译并运行程序:
```
make
./main
```
输出结果为:
```
The gcd of 12 and 18 is 6
```
相关推荐
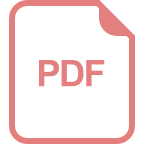














