<?php // 连接数据库 $conn = new mysqli("localhost", "root", "123456", "wyya"); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 查询数据 $tables = array("one_list", "two_list", "three_list", "four_list", "five_list"); $data = array(); foreach ($tables as $table) { $sql = "SELECT * FROM " . $table; $result = mysqli_query($conn, $sql); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $row['语种'] = substr($table, 0, -5); // 获取语种名称 $data[] = $row; } } } // 渲染 HTML 模板 ?> <!DOCTYPE html> <html> <head> <title>网易云音乐歌单</title> <style> table { border-collapse: collapse; width: 100%; } th, td { text-align: left; padding: 8px; } tr:nth-child(even) { background-color: #f2f2f2; } th { background-color: #4CAF50; color: white; } .language-button { background-color: #f2f2f2; padding: 8px 16px; border: none; cursor: pointer; display: inline-block; margin-right: 10px; } .chinese { color: #e60000; } .english { color: #0066cc; } .japanese { color: #ff9900; } .korean { color: #3385ff; } .cantonese { color: #009933; } </style> </head> <body> <div> <h2>网易云音乐歌单</h2> <div> <form method="get" action=""> <input type="hidden" name="table" value="<?php echo $tables[0]; ?>"> <button type="submit" class="language-button chinese">华语</button> </form> <form method="get" action=""> <input type="hidden" name="table" value="<?php echo $tables[1]; ?>"> <button type="submit" class="language-button english">英语</button> </form> <form method="get" action=""> <input type="hidden" name="table" value="<?php echo $tables[2]; ?>"> <button type="submit" class="language-button japanese">日语</button> </form> <form method="get" action=""> <input type="hidden" name="table" value="<?php echo $tables[3]; ?>"> <button type="submit" class="language-button korean">韩语</button> </form> <form method="get" action=""> <input type="hidden" name="table" value="<?php echo $tables[4]; ?>"> <button type="submit" class="language-button cantonese">粤语</button> </form> </div> </div> <?php if (!empty($data)) { ?> <table> <tbody> <tr> <th>歌单名</th> <th>歌单地址</th> <th>歌曲量</th> <th>播放量</th> <th>收藏量</th> <th>评论量</th> <th>分享量</th> <th>创建者</th> <th>创建时间</th> </tr> <?php foreach ($data as $row) { ?> <tr> <td><?php echo $row['歌单名']; ?></td> <td><a href="<?php echo $row['歌单地址']; ?>" target="_blank"><?php echo $row['歌单地址']; ?></a></td> <td><?php echo $row['歌曲量']; ?></td> <td><?php echo $row['播放量']; ?></td> <td><?php echo $row['收藏量']; ?></td> <td><?php echo $row['评论量']; ?></td> <td><?php echo $row['分享量']; ?></td> <td><?php echo $row['创建者']; ?></td> <td><?php echo $row['创建时间']; ?></td> </tr> <?php } ?> </tbody> </table> <?php } else { ?> <p>暂无数据</p> <?php } ?> </body> </html> <?php // 关闭连接 $conn->close()0>修改代码,通过点击按钮展示出对应的单个歌单
时间: 2023-08-14 16:26:23 浏览: 84
好的,您可以将代码修改为以下内容:
```php
<?php
// 连接数据库
$conn = new mysqli("localhost", "root", "123456", "wyya");
// 检查连接是否成功
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
$tables = array("one_list", "two_list", "three_list", "four_list", "five_list");
$current_table = isset($_GET['table']) ? $_GET['table'] : $tables[0]; // 获取当前语种
// 查询数据
$sql = "SELECT * FROM " . $current_table;
$result = mysqli_query($conn, $sql);
$data = array();
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
$row['语种'] = substr($current_table, 0, -5); // 获取语种名称
$data[] = $row;
}
}
// 渲染 HTML 模板
?>
<!DOCTYPE html>
<html>
<head>
<title>网易云音乐歌单</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
th {
background-color: #4CAF50;
color: white;
}
.language-button {
background-color: #f2f2f2;
padding: 8px 16px;
border: none;
cursor: pointer;
display: inline-block;
margin-right: 10px;
}
.chinese {
color: #e60000;
}
.english {
color: #0066cc;
}
.japanese {
color: #ff9900;
}
.korean {
color: #3385ff;
}
.cantonese {
color: #009933;
}
</style>
</head>
<body>
<div>
<h2>网易云音乐歌单</h2>
<div>
<?php foreach ($tables as $table) { ?>
<form method="get" action="">
<input type="hidden" name="table" value="<?php echo $table; ?>">
<button type="submit" class="language-button <?php echo substr($table, 0, -5); ?>" <?php echo $table == $current_table ? 'disabled' : ''; ?>><?php echo substr($table, 0, -5); ?></button>
</form>
<?php } ?>
</div>
</div>
<?php if (!empty($data)) { ?>
<table>
<tbody>
<tr>
<th>歌单名</th>
<th>歌单地址</th>
<th>歌曲量</th>
<th>播放量</th>
<th>收藏量</th>
<th>评论量</th>
<th>分享量</th>
<th>创建者</th>
<th>创建时间</th>
</tr>
<?php foreach ($data as $row) { ?>
<tr>
<td><?php echo $row['歌单名']; ?></td>
<td><a href="<?php echo $row['歌单地址']; ?>" target="_blank"><?php echo $row['歌单地址']; ?></a></td>
<td><?php echo $row['歌曲量']; ?></td>
<td><?php echo $row['播放量']; ?></td>
<td><?php echo $row['收藏量']; ?></td>
<td><?php echo $row['评论量']; ?></td>
<td><?php echo $row['分享量']; ?></td>
<td><?php echo $row['创建者']; ?></td>
<td><?php echo $row['创建时间']; ?></td>
</tr>
<?php } ?>
</tbody>
</table>
<?php } else { ?>
<p>暂无数据</p>
<?php } ?>
</body>
</html>
<?php
// 关闭连接
$conn->close();
?>
```
修改后,您可以点击对应语种的按钮,展示出该语种对应的单个歌单。
相关推荐
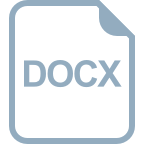
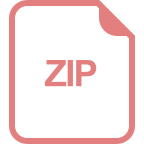
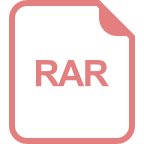


检查这段代码的错误并修改实现与bookshelf.php的跳转<?php //连接数据库 $servername = "127.0.0.12"; $username = "roots"; $password = "1234root"; $dbname = "zkw"; $conn = mysqli_connect($servername, $username, $password, $dbname); if (!$conn) { die("连接失败: " . mysqli_connect_error()); } //查询图书分类 $sql = "SELECT * FROM categories"; $result = mysqli_query($conn, $sql); mysqli_close($conn); //跳转到bookshelf.php页面 header("Location: bookshelf.php"); ?> <!DOCTYPE html> <html> <head> <title>图书分类</title> </head> <body> 图书分类 <?php while ($row = mysqli_fetch_assoc($result)) { ?> "><?php echo $row["name"]; ?> <?php } ?> </body> </html>

<?php // 连接数据库 $conn = new mysqli("localhost", "root", "123456", "wyya"); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 查询五个歌单的表 $tables = array(); $result = mysqli_query($conn, "SHOW TABLES LIKE '%_list'"); if ($result->num_rows > 0) { while ($row = mysqli_fetch_array($result)) { $tables[] = $row[0]; } } // 获取选中的歌单表 $tableName = isset($_GET["table"]) ? $_GET["table"] : ""; $data = array(); if (!empty($tableName)) { $result = mysqli_query($conn, "SELECT * FROM $tableName"); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $data[] = $row; } } } ?> <!DOCTYPE html> <html> <head> <title>网易云音乐歌单</title> <style> table { border-collapse: collapse; width: 100%; } th, td { text-align: left; padding: 8px; } tr:nth-child(even) { background-color: #f2f2f2; } th { background-color: #4CAF50; color: white; } </style> </head> <body> 网易云音乐歌单 华语 欧美 日语 韩语 粤语 <?php if (!empty($tableName)) { ?> <?php $columns = mysqli_query($conn, "SHOW COLUMNS FROM $tableName"); if ($columns->num_rows > 0) { while ($column = mysqli_fetch_array($columns)) { ?> <?php echo $column["Field"]; ?> <?php } } ?> <?php foreach ($data as $row) { ?> <?php foreach ($row as $value) { ?> <?php echo $value; ?> <?php } ?> <?php } ?> <?php } ?> </body> </html> <?php // 关闭连接 $conn->close(); ?>修改代码,将华语,欧美,日语,韩语,粤语放入一个命名为分类的菜单中,实现点击菜单后点击华语,欧美,日语,韩语,粤语再跳转




检查这段代码的错误并修改实现与book.php的跳转<?php header("Location: book.php"); //连接数据库 $servername = "127.0.0.12"; $username = "roots"; $password = "1234root"; $dbname = "zkw"; $conn = mysqli_connect($servername, $username, $password, $dbname); if (!$conn) { die("连接失败: " . mysqli_connect_error()); } //查询图书 $id = $_GET["id"]; $sql = "SELECT * FROM books WHERE id=$id"; $result = mysqli_query($conn, $sql); $row = mysqli_fetch_assoc($result); //检查用户是否登录 session_start(); if (!isset($_SESSION["username"])) { $bookshelf_text = "登录后可以添加到书架"; } else { $username = $_SESSION["username"]; $sql = "SELECT * FROM bookshelf WHERE username='$username' AND book_id=$id"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { $bookshelf_text = "从书架中移除"; } else { $bookshelf_text = "添加到书架"; } } mysqli_close($conn); ?> <!DOCTYPE html> <html> <head> <title><?php echo $row["name"]; ?></title> </head> <body> <?php echo $row["name"]; ?> <?php echo $row["description"]; ?> <?php echo $bookshelf_text; ?> </body> </html>







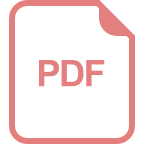
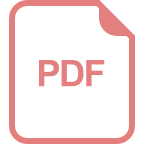