用c语言编程实现建立一个顺序表并在此表中插入一个元素和删除一个元素
时间: 2024-10-14 18:13:15 浏览: 5
在C语言中,我们可以创建一个顺序表(也称为数组列表)通过动态分配内存来存储数据。下面是一个简单的例子,展示如何实现顺序表的插入和删除操作:
首先,我们定义一个结构体(struct)来表示表中的元素:
```c
typedef struct {
int data; // 表示整数的数据域
struct Node* next; // 指向下一个元素的指针
} Node;
```
然后定义一个顺序表的全局变量和相关的函数:
```c
Node* head = NULL; // 初始头节点
// 插入元素
void insert(int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 动态分配空间
if (newNode != NULL) {
newNode->data = value;
newNode->next = head;
head = newNode;
printf("Element %d inserted successfully.\n", value);
} else {
printf("Memory allocation failed.\n");
}
}
// 删除元素
void delete(int target) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* current = head;
while (current != NULL && current->data != target) {
current = current->next;
}
if (current != NULL) {
Node* temp = current;
current = current->next;
free(temp); // 释放当前节点的内存
printf("Element %d deleted successfully.\n", target);
} else {
printf("Element not found in the list.\n");
}
}
```
相关推荐
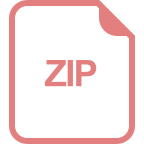
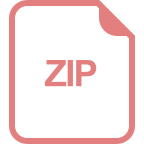
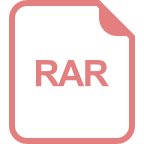


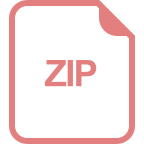
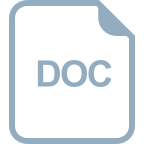



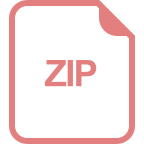
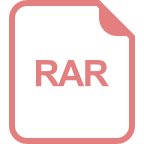
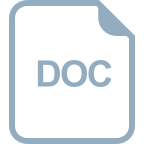
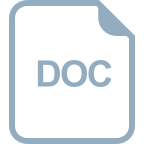
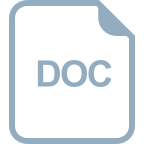
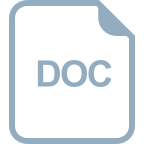
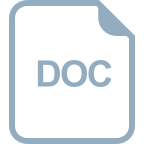
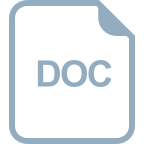
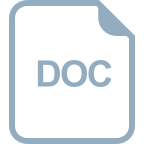