用C语言写出在单链表上结点的数据域为X的结点之前插入一个新结点的程序
时间: 2024-10-16 18:12:59 浏览: 43
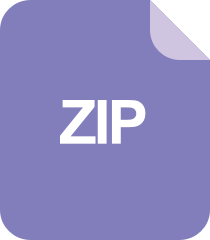
链表-使用C语言实现带头结点的单链表.zip
在C语言中,为了在单链表上结点的数据域为`X`的结点之前插入一个新节点,你需要首先定义链表结构、创建新节点、找到数据域为`X`的位置以及插入新节点。这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} Node;
// 插入新节点的函数
void insertBeforeNode(Node** head, int X, int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value; // 设置新节点的数据
newNode->next = *head; // 新节点指向头节点
Node* current = *head;
while (current != NULL && current->data != X) { // 找到X前的节点
current = current->next;
}
if (current == NULL) { // 如果未找到X,说明已经是链表头部
printf("No node with data %d found.\n", X);
} else {
current->prev = newNode; // 新节点的前一个节点设置为当前节点
*head = newNode; // 更新头节点
}
}
int main() {
Node* head = NULL; // 初始化链表为空
// 添加一些数据节点
insertBeforeNode(&head, 5, 3); // 在数据为5的节点之前插入3
insertBeforeNode(&head, 5, 4); // 在数据为5的节点之前插入4
// 显示链表(假设其他节点数据)
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
return 0;
}
```
阅读全文
相关推荐
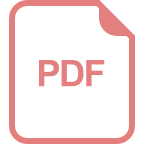
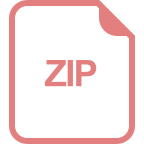















