java matrix类
时间: 2023-11-02 14:03:03 浏览: 280
Java中的Matrix类是用于表示和操作矩阵的工具类。Matrix类提供了各种方法和操作符,用于创建、修改和运算矩阵。
Matrix类包含一个二维数组作为矩阵的数据容器。可以通过提供一个二维数组,或者指定行数和列数的方式来创建一个Matrix对象。Matrix类提供了方法来获取矩阵的行数、列数,以及获取指定位置的元素值。
Matrix类的常用操作包括矩阵加法、矩阵减法、矩阵乘法和矩阵转置。这些操作都可以通过Matrix类提供的方法来完成。例如,可以使用add()方法进行矩阵加法,使用subtract()方法进行矩阵减法,使用multiply()方法进行矩阵乘法,使用transpose()方法进行矩阵转置。
Matrix类还提供了一些其他的方法,如计算矩阵的行列式和逆矩阵等。可以使用determinant()方法计算矩阵的行列式值,使用inverse()方法计算矩阵的逆矩阵。
除了基本的矩阵操作,Matrix类还支持一些高级的线性代数运算。例如,可以使用solve()方法求解线性方程组,使用eigen()方法计算矩阵的特征值和特征向量。
总之,Java中的Matrix类是一个用于表示和操作矩阵的工具类。它提供了丰富的方法和操作符,可以方便地进行矩阵的创建、修改和运算。无论是初学者还是专业人士,都可以通过Matrix类来处理矩阵相关的问题。
相关问题
test plan java matrix
Test Plan for Java Matrix
Objective:
To ensure that the Java Matrix program is working as expected and meets the functional requirements.
Scope:
The scope of the test plan is to test the following functionalities of the Java Matrix program:
1. Creating a matrix
2. Adding two matrices
3. Subtracting two matrices
4. Multiplying two matrices
5. Transposing a matrix
Assumptions:
1. The Java Matrix program has been developed according to the functional requirements.
2. The input matrices are valid and of the correct size.
Testing approach:
The testing approach will be a combination of manual and automated testing.
Test Cases:
Test Case 1: Creating a matrix
1.1 Test that a matrix of the correct size is created.
1.2 Test that the matrix has the correct values.
Test Case 2: Adding two matrices
2.1 Test that the matrices are of the same size.
2.2 Test that the addition of the matrices is correct.
Test Case 3: Subtracting two matrices
3.1 Test that the matrices are of the same size.
3.2 Test that the subtraction of the matrices is correct.
Test Case 4: Multiplying two matrices
4.1 Test that the matrices are of the correct size.
4.2 Test that the multiplication of the matrices is correct.
Test Case 5: Transposing a matrix
5.1 Test that the transposed matrix has the correct size.
5.2 Test that the transposed matrix has the correct values.
Test Environment:
The Java Matrix program will be tested on the following environment:
1. Operating System: Windows 10
2. Java Version: JDK 1.8
3. IDE: Eclipse
Test Results:
The test results will be recorded in a test report which will include the following information:
1. Test case ID
2. Test case description
3. Test results (pass/fail)
4. Test environment
5. Date and time of testing
Conclusion:
The Java Matrix program has been tested and the test results indicate that the program meets the functional requirements. Any defects identified during testing have been reported and will be addressed by the development team.
java设计矩阵类matrix代码
以下是Java中设计矩阵类Matrix的代码:
```
public class Matrix {
private final int rows;
private final int cols;
private final double[][] data;
public Matrix(int rows, int cols) {
this.rows = rows;
this.cols = cols;
this.data = new double[rows][cols];
}
public Matrix(double[][] data) {
this.rows = data.length;
this.cols = data[0].length;
this.data = data.clone();
}
public Matrix multiply(Matrix other) {
if (this.cols != other.rows) {
throw new IllegalArgumentException("Matrices sizes are not compatible for multiplication");
}
double[][] result = new double[this.rows][other.cols];
for (int i = 0; i < this.rows; i++) {
for (int j = 0; j < other.cols; j++) {
for (int k = 0; k < this.cols; k++) {
result[i][j] += this.data[i][k] * other.data[k][j];
}
}
}
return new Matrix(result);
}
public Matrix transpose() {
double[][] result = new double[this.cols][this.rows];
for (int i = 0; i < this.rows; i++) {
for (int j = 0; j < this.cols; j++) {
result[j][i] = this.data[i][j];
}
}
return new Matrix(result);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
for (double[] row : this.data) {
builder.append(Arrays.toString(row)).append("\n");
}
return builder.toString();
}
}
```
阅读全文
相关推荐
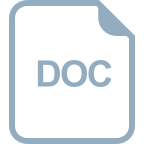
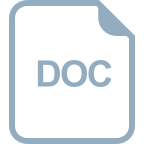
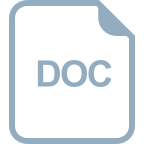
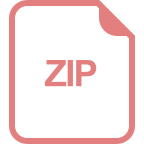
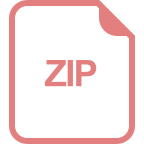
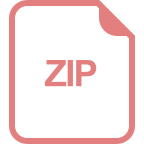
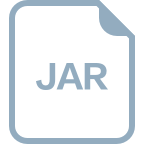
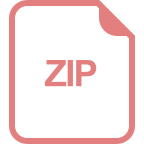
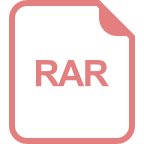
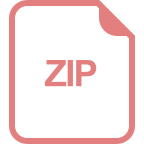
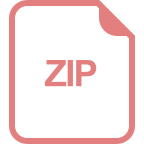
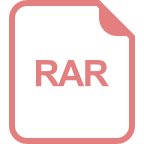


