超市商品管理系统c++
时间: 2023-07-19 18:14:54 浏览: 131
好的,您的问题是关于超市商品管理系统的C++实现。那么,您需要了解以下几点:
1. 确定系统功能:超市商品管理系统需要支持哪些功能,如商品管理、库存管理、销售管理、会员管理等。
2. 设计系统结构:确定系统的模块和组件,如UI界面、商品信息类、库存信息类、销售信息类、会员信息类等。
3. 编写代码:根据系统结构设计,分别编写各个模块和组件的代码,并进行集成测试。
以下是一个简单的C++超市商品管理系统的实现示例:
```
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Goods {
private:
string name;
double price;
int count;
public:
Goods(string n, double p, int c) {
name = n;
price = p;
count = c;
}
string GetName() {
return name;
}
double GetPrice() {
return price;
}
int GetCount() {
return count;
}
void SetCount(int c) {
count = c;
}
};
class Store {
private:
vector<Goods> goodsList;
public:
void AddGoods(string name, double price, int count) {
Goods newGoods(name, price, count);
goodsList.push_back(newGoods);
}
void DeleteGoods(int index) {
goodsList.erase(goodsList.begin() + index);
}
void ShowGoodsList() {
cout << "商品列表:" << endl;
for (int i = 0; i < goodsList.size(); i++) {
cout << i << ". " << goodsList[i].GetName() << " 价格:" << goodsList[i].GetPrice() << " 数量:" << goodsList[i].GetCount() << endl;
}
}
};
int main() {
Store store;
store.AddGoods("可乐", 2.5, 100);
store.AddGoods("雪碧", 2.5, 100);
store.AddGoods("芬达", 2.5, 100);
store.ShowGoodsList();
return 0;
}
```
以上代码实现了一个简单的超市商品管理系统,包括商品类和超市类,可以添加商品、删除商品和展示商品列表。您可以根据需求进一步完善系统功能和代码设计。
阅读全文
相关推荐



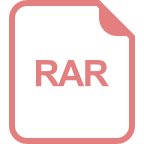


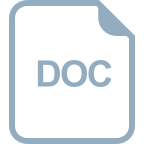




