通过vue通过bootstrap框架写一个商品列表界面,能通过商品id,商品名称查询,表内容包括商品id,商品名称,商品种类,有效期
时间: 2024-05-04 17:18:57 浏览: 120
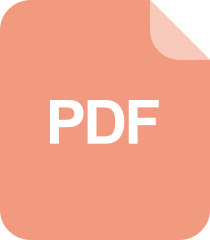
使用vue框架 Ajax获取数据列表并用BootStrap显示出来
首先,你需要在Vue项目中安装Bootstrap框架,可以使用以下命令:
```
npm install bootstrap
```
接下来,你需要在Vue组件中引入Bootstrap样式表和JavaScript文件:
```html
<template>
<div>
<div class="form-group">
<label for="inputId">商品ID</label>
<input type="text" class="form-control" id="inputId" v-model="searchId">
</div>
<div class="form-group">
<label for="inputName">商品名称</label>
<input type="text" class="form-control" id="inputName" v-model="searchName">
</div>
<button class="btn btn-primary" @click="search">查询</button>
<table class="table">
<thead>
<tr>
<th>商品ID</th>
<th>商品名称</th>
<th>商品种类</th>
<th>有效期</th>
</tr>
</thead>
<tbody>
<tr v-for="item in items" :key="item.id">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.category }}</td>
<td>{{ item.expiry }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
searchId: '',
searchName: '',
items: []
}
},
methods: {
search() {
axios.get(`/api/items?id=${this.searchId}&name=${this.searchName}`)
.then(response => {
this.items = response.data;
})
.catch(error => {
console.error(error);
});
}
}
}
</script>
```
在上面的代码中,我们使用了Bootstrap的表单、按钮和表格样式,并使用了Axios库来发送查询请求。在`data`中定义了`searchId`、`searchName`和`items`三个变量,分别表示查询的商品ID、商品名称和查询结果。在`methods`中定义了`search`方法,用于发送查询请求并更新查询结果。`v-model`指令用于实现双向数据绑定,当用户在输入框中输入内容时,Vue会自动更新相应的变量。`v-for`指令用于遍历查询结果并渲染到表格中。
最后,你需要在服务器端实现查询接口,例如使用Express框架:
```javascript
const express = require('express');
const app = express();
const items = [
{ id: 1, name: '商品1', category: '类别1', expiry: '2022-01-01' },
{ id: 2, name: '商品2', category: '类别2', expiry: '2022-02-01' },
{ id: 3, name: '商品3', category: '类别1', expiry: '2022-03-01' },
{ id: 4, name: '商品4', category: '类别2', expiry: '2022-04-01' }
];
app.get('/api/items', (req, res) => {
const id = req.query.id;
const name = req.query.name;
let result = items;
if (id) {
result = result.filter(item => item.id.toString().includes(id));
}
if (name) {
result = result.filter(item => item.name.includes(name));
}
res.send(result);
});
app.listen(3000, () => {
console.log('Server is listening on port 3000');
});
```
在上面的代码中,我们使用了一个静态的商品列表,并实现了一个查询接口`/api/items`,接受两个查询参数`id`和`name`,分别表示商品ID和商品名称。我们使用`filter`方法对商品列表进行过滤,并返回符合条件的结果。最后,我们使用`send`方法将查询结果发送给客户端。
阅读全文
相关推荐
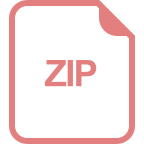
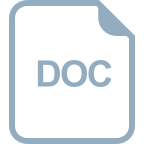
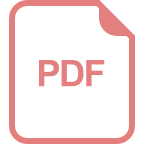
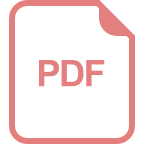
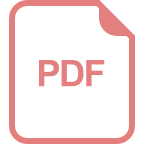
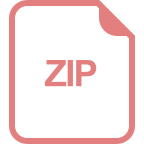
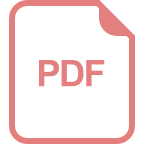
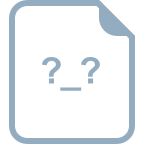
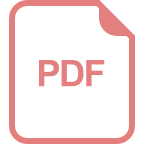
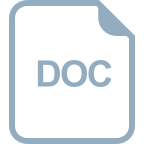
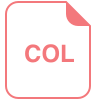




