springboot项目批量删除的相关代码
时间: 2023-05-26 22:07:28 浏览: 46
以下是一个Spring Boot项目中实现批量删除的示例代码:
1. 定义实体类(例如:User.java)
```java
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
// getters and setters
}
```
2. 创建Repository(例如:UserRepository.java)
```java
public interface UserRepository extends JpaRepository<User, Long> {
// 自定义方法,根据id批量删除用户
void deleteByIdIn(List<Long> ids);
}
```
3. 创建Service(例如:UserService.java)
```java
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public void deleteUsers(List<Long> ids) {
userRepository.deleteByIdIn(ids);
}
}
```
4. 创建Controller(例如:UserController.java)
```java
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@DeleteMapping
public ResponseEntity<Object> deleteUsers(@RequestBody List<Long> ids) {
try {
userService.deleteUsers(ids);
return new ResponseEntity<>("Users have been deleted successfully.", HttpStatus.OK);
} catch (Exception e) {
return new ResponseEntity<>(e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
```
在以上示例中,我们定义了一个用户实体类,一个用户Repository和UserService,以及一个UserController。在UserService中,我们定义了一个方法,该方法使用批量删除的方式删除用户。在UserController中,我们使用@DeleteMapping注释来定义一个HTTP DELETE请求,这个请求接受一个包含用户id的列表作为请求体,并使用UserService来执行批量删除操作。如果删除成功,返回一个HTTP 200 OK响应,否则返回一个HTTP 500错误响应。
相关推荐
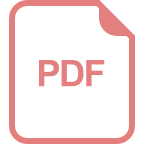
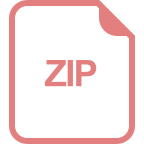
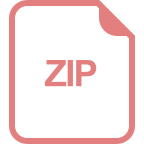














