利用RSA算法加解密学号,JAVA代码实现
时间: 2023-12-01 21:56:48 浏览: 81
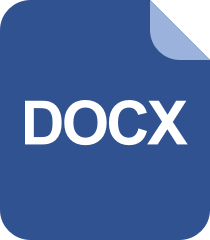
RSA加解密算法java实现
好的,下面是利用RSA算法加解密学号的JAVA代码实现:
```
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import javax.crypto.Cipher;
import java.util.Base64;
public class RSAEncryptDecryptExample {
public static void main(String[] args) throws Exception {
// 生成公钥和私钥
KeyPair keyPair = KeyPairGenerator.getInstance("RSA").generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 用公钥加密学号
String studentId = "202100000000";
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedStudentId = cipher.doFinal(studentId.getBytes());
String encryptedStudentIdStr = Base64.getEncoder().encodeToString(encryptedStudentId);
System.out.println("加密后的学号:" + encryptedStudentIdStr);
// 用私钥解密学号
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedStudentId = cipher.doFinal(Base64.getDecoder().decode(encryptedStudentIdStr));
String decryptedStudentIdStr = new String(decryptedStudentId);
System.out.println("解密后的学号:" + decryptedStudentIdStr);
}
}
```
在上面的程序中,我们首先用 `KeyPairGenerator` 生成公钥和私钥,然后用公钥加密学号,最后用私钥解密学号。加密和解密的过程中,我们使用 `Cipher` 类来实现,具体来说,我们通过调用 `Cipher.init()` 方法来初始化加密/解密模式和密钥,然后通过 `Cipher.doFinal()` 方法来进行加密/解密操作。在加密和解密的过程中,我们还使用了 `Base64` 类来将二进制数据转换成字符串,以便于输出和传输。
需要注意的是,上述代码仅为示例代码,实际加密和解密操作中,我们需要采用更严格的密钥管理和身份验证机制,以确保加密和解密的安全性。
阅读全文
相关推荐
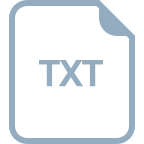
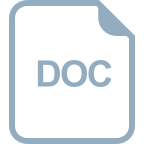
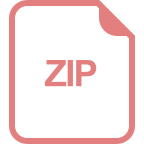
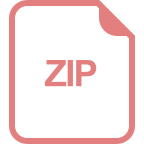
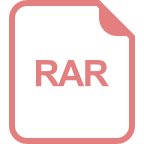
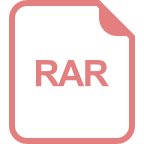
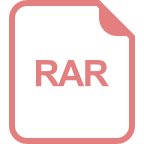
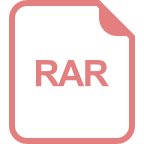
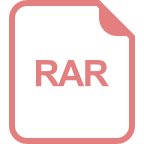
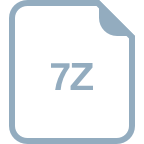
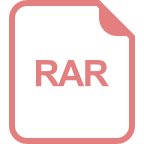